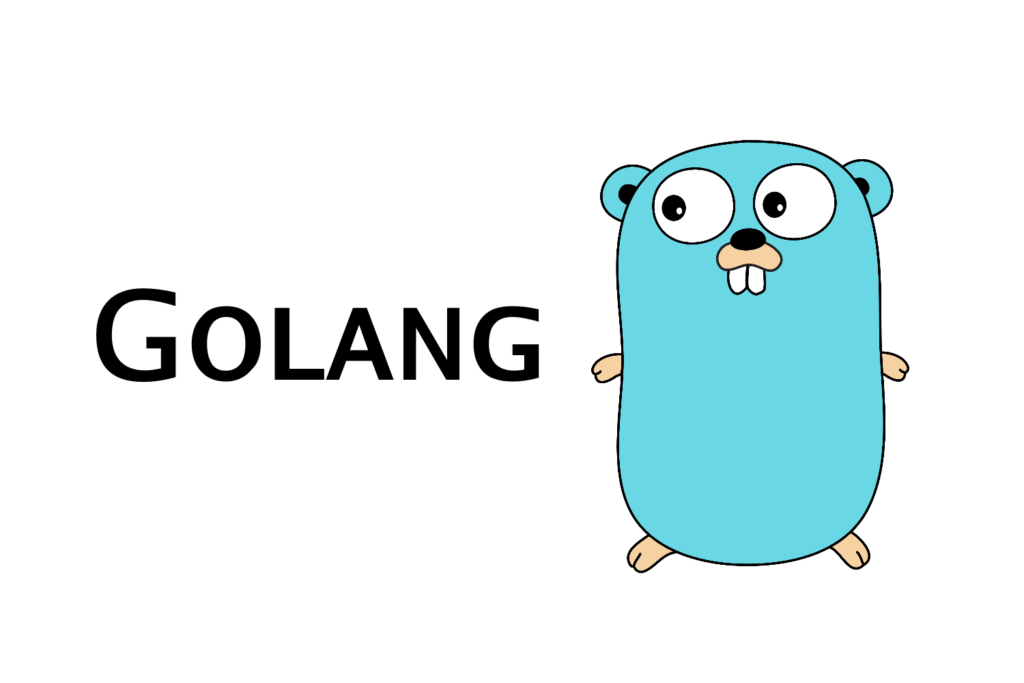
So, I've been tooling around on some free online resources lately, and...
Even though I said Rust is the programming language for the next 40 years due to its ability to enable programmers to write fast and safe "go fast" code at a low level, it seems that human society has decieded to build a bunch of useful things in a garbage-collected language simmiliar to Java from Google called Go(lang) (those &%#@^*$).
It's interesting how quickly Go has gained popularity, and how pervasive it is amongst the new stack sort of tools that are starting to take over software and enterprise computing patterns (i.e. Docker, Kubernetes, containers, hip new tech, code that mows your lawn and walks your dog, etc.).
So why is it so popular, even though it's supposedly an objectively "bad" language?
My uninformed guess is that it's garbage collected like Java (where you don't have to hand back resources to the machine, you just stop using them and it automatically comes around and "picks up the trash"), so it's open season for anyone who's used to working with higher level languages to get started working with. In addition, I would guess that anyone who's taken intro Computer Science classes (which are often taught in Java) could hop on over and start working with Go.
Also, it seems Go seems to have some cool features that make it really useful for networked and multi-threaded code (where multiple tracks of execution are happening at once on one or more CPU cores).
With all of this being said, it's probably a decent idea to learn a little bit of Go given how powerful and popular it is. For either reading code from the plethora of open source tools, or modifying or making your own tools, knowing a bit of Go could be useful.
When in Rome, do as the Romans and all that...
You may notice today's blog post is of a different format than usual. That's because I've decided to write it using markdown on my Github so that I could write this diatribe, and so that I could include the laundry list of (useful?) tools that humans have decided to write in Go below.
Enjoy!
These sites provide indexes and search engines for Go packages:
- Awesome Go @LibHunt - Your go-to Go Toolbox.
- godoc.org - A documentation browser for any Go open source package.
- go-hardware - Curated list of resources for using Go on non-standard hardware.
- go-patterns - Commonly used patterns and idioms for Go.
- gopm.io - Download Go packages by version
- go-search - Search engine dedicated to Go projects and source.
- gowalker - API documentation generator and search.
- Go Report Card - Code quality summaries for any Go project.
- Sourcegraph - Source indexing, analysis and search.
- Codeseek.com - Custom search engine with a wealth of Go blogs.
If you find a project in this list that is dead or broken, please either mark it as such or mention it in the #go-nuts IRC channel.
- Astronomy
- Build Tools
- Caching
- Cloud Computing
- Command-line Option Parsers
- Command-line Tools
- Compression
- Concurrency and Goroutines
- Configuration File Parsers
- Console User Interface
- Continuous Integration
- Cryptocurrency
- Cryptography
- Databases
- Data Processing
- Data Structures
- Date
- Development Tools
- Distributed/Grid Computing
- Documentation
- Editors
- Encodings and Character Sets
- Error handling
- File Systems
- Games
- GIS
- Go Implementations
- Graphics and Audio
- GUIs and Widget Toolkits
- Hardware
- Language and Linguistics
- Logging & Monitoring
- Machine Learning & AI
- Mathematics
- Microservices
- Miscellaneous
- Music
- Networking
- DNS
- FTP
- HTTP
- IMAP
- Instant Messaging
- NNTP
- Protocol Buffers
- rsync
- Telnet
- VNC
- Websockets
- ZeroMQ
- Misc Networking
- Operating System Interfaces
- Other Random Toys, Experiments and Example Code
- P2P and File Sharing
- Programming
- Resource Embedding
- RPC
- Scanner and Parser Generators
- Security
- Simulation Modeling
- Sorting
- Source Code Management
- Storage
- Strings and Text
- Testing
- Transpiler
- Unix
- Unsorted
- Validation
- Version Control
- Virtual Machines and Languages
- Web Applications
- Web Libraries
- Windows
- go-fits - FITS (Flexible Image Transport System) format image and data reader
- astrogo/fitsio - Pure Go FITS (Flexible Image Transport System) format image and data reader/writer
- cosmo - Cosmological distance and time calculations for common cosmologies (Friedmann-Lemaître-Robertson-Walker metric).
- gonova - A wrapper for libnova -- Celestial Mechanics, Astrometry and Astrodynamics Library
- meeus - Implementation of "Astronomical Algorithms" by Jean Meeus
- novas - Interface to the Naval Observatory Vector Astrometry Software (NOVAS)
- utdfgo - Spacecraft UTDF Packet Reader and Decoder
- beku - A library and program to manage packages in user's environment (GOPATH or vendor directory)
- colorgo - Colorize go build output
- dogo - Monitoring changes in the source file and automatically compile and run (restart)
- fileembed-go - This is a command-line utility to take a number of source files, and embed them into a Go package
- gb - A(nother) build tool for go, with an emphasis on multi-package projects
- gg - A tiny multi golang projects env/make management tool.
- GG - A build tool for Go in Go
- godag - A frontend to the Go compiler collection
- goenv - goenv provides Go version and Go workspace management tools
- gopei - Simple Go compiler and LiteIDE installer for Unix/Linux that adds many features like github support and presenter.
- go-pkg-config - lightweight clone of pkg-config
- goscons - Another set of SCons builders for Go
- go-server - Agile server framework.
- gotgo - An experimental preprocessor to implement 'generics'
- gows - Go workspace manager
- goxc - A build tool with a focus on cross-compiling, packaging, versioning and distribution
- GVM - GVM provides an interface to manage Go versions
- Realize - A Go build system with file watchers, output streams and live reload. Run, build and watch file changes with custom paths.
- SCons Go Tools - A collection of builders that makes it easy to compile Go projects in SCons
- Task - A task runner / simple alternative to Make
- cache - LevelDB style LRU cache for Go, support non GC object cache.
- cache2go - Concurrency-safe caching library with expiration capabilities and access counters
- go-cache - An in-memory key:value store/cache (similar to Memcached) library for Go, suitable for single-machine applications
- golibs/cache - A tiny cache package
- gomemcached - A memcached server in go
- gomemcache - a memcached client
- go-slab - Slab allocator for go.
- groupcache - Caching and cache-filling library, intended as a replacement for memcached in many cases
- libmemcache - Fast client and server libraries speaking memcache protocol
- memcached-bench - Benchmark tool for memcache servers
- memcached - Fast memcache server, which supports persistence and cache sizes exceeding available RAM
- memcache - go memcached client, forked from YouTube Vitess
- rend - A memcached proxy that manages data chunking and L1/L2 caches
- YBC bindings - Bindings for YBC library providing API for fast in-process blob cache
- LXD Daemon based on liblxc offering a REST API to manage containers
- Docker - The Linux container runtime. Developed by dotCloud.
- Enduro/X ASG Application Server for Go. Provides application server and middleware facilities for distributed transaction processing. Supports microservices based application architecture. Developed by ATR Baltic.
- Kubernetes - Container Cluster Manager from Google.
- flamingo - A Lightweight Cloud Instance Contextualizer.
- gocircuit - A distributed operating system that sits on top of the traditional OS on multiple machines in a datacenter deployment. It provides a clean and uniform abstraction for treating an entire hardware cluster as a single, monolithic compute resource. Developed by Tumblr.
- gosync - A package for syncing data to and from S3.
- juju - Orchestration tool (deployment, configuration and lifecycle management), developed by Canonical.
- mgmt - Next Generation Configuration Management tool (parallel, event driven, distributed system) developed by @purpleidea, (a Red Hat employee) and the mgmt community.
- rclone - "rsync for cloud storage" - Google Drive, Amazon Drive, S3, Dropbox, Backblaze B2, One Drive, Swift, Hubic, Cloudfiles, Google Cloud Storage, Yandex Files
- ShipBuilder - ShipBuilder is a minimalist open source platform as a service, developed by Jay Taylor.
- swift - Go language interface to Swift / Openstack Object Storage / Rackspace cloud files
- Tsuru - Tsuru is an open source polyglot cloud computing platform as a service (PaaS), developed by Globo.com.
- aws-sdk-go - AWS SDK for the Go programming language.
- argcfg - Use reflection to populate fields in a struct from command line arguments
- autoflags - Populate go command line app flags from config struct
- cobra - A commander for modern go CLI interactions supporting commands & POSIX/GNU flags
- command - Add subcommands to your CLI, provides help and usage guide.
- docopt.go - An implementation of docopt in the Go programming language.
- flaq - Command-line options parsing library, POSIX/GNU compliant, supports struct tags as well as the Go's flag approach.
- getopt - full featured traditional (BSD/POSIX getopt) option parsing in Go style
- getopt - Yet Another getopt Library for Go. This one is like Python's.
- gnuflag - GNU-compatible flag parsing; substantially compatible with flag.
- go-commander - Simplify the creation of command line interfaces for Go, with commands and sub-commands, with argument checks and contextual usage help. Forked from the "go" tool code.
- go-flags - command line option parser for go
- goopt - a getopt clone to parse command-line flags
- go-options - A command line parsing library for Go
- options - Self documenting CLI options parser
- opts.go - lightweight POSIX- and GNU- style option parsing
- pflag - Drop-in replacement for Go's flag package, implementing POSIX/GNU-style --flags.
- subcommands - A concurrent, unit tested, subcommand library
- uggo - Yet another option parser offering gnu-like option parsing. This one wraps (embeds) flagset. It also offers rudimentary pipe-detection (commands like ls behave differently when being piped to).
- writ - A flexible option parser with thorough test coverage. It's meant to "just work" and stay out of the way.
- cli - A Go library for implementing command-line interfaces.
mellium.im/cli
— A library for parsing modern CLI apps including subcommands that may have their own flags and a built in help system. Designed to use a minimal API.- cmdline - A simple parser with support for short and long options, default values, arguments and subcommands.
- go-getoptions - Go option parser inspired on the flexibility of Perl’s GetOpt::Long.
- awless - A Mighty command-line interface for Amazon Web Services (AWS).
- boilr - A blazing fast CLI tool for creating projects from boilerplate templates.
- coshell - A drop-in replacement for GNU 'parallel'.
- DevTodo2 - A small command-line per-project task list manager.
- dsio - Command line tool for Google Cloud Datastore.
- enumeration - Easy enumeration code generation.
- fzf - A command-line fuzzy finder
- gich - A cross platform which utility written in Go
- git-time-metric - Simple, seamless, lightweight time tracking for Git
- gister - Manage your github gists from the command-line
- gmail2go - Simple gmail multiple accounts cli mail checker
- gocreate - Command line utility that create files from templates.
- godocdoc - Start godoc and open it in your browser to the project in the current directory.
- gojson - Command-line tool for manipulating JSON for use in developing Go code.
- gopass - Command line password manager with git syncing capabilities
- GoPasswordCreator - A small tool, which creates random passwords
- GoNote - Command line SimpleNote client.
- Grozilla - File downloader utility with resume capability.
- jsonpp - A fast command line JSON pretty printer.
- lsp - A human-friendlier alternative to
ls
- ltst - View the latest news of your choosing right in your terminal
- passhash - Command-line utility to create secure password hashes
- passman - A command-line password manager
- pdfcpu - PDF processor.
- pjs - Pretty print and search through JSON data structures fast.
- project - Very simple CLI tool to setup new projects from boilerplate templates.
- redis-view - A tree like tool help you explore data structures in your redis server
- remote-torrent - A simple tool for downloading Torrent remotely and retrieving files back over HTTP at full speed without ISP Torrent limitation
- restic - A fast, efficient and secure backup program
- runtemplate - A very simple command-line tool for executing Go templates, useful for use with
go generate
. - runtemplate - A simple tool for executing Go templates to support generating Go code for your types.
- sift - A fast and powerful open source alternative to
grep
- tecla - Command-line editing library
- wlog - A simple logging interface that supports cross-platform color and concurrency.
- wmenu - An easy to use menu structure for cli applications that prompts users to make choices.
- comb-go - A CLI tool implemented by Golang to manage CloudComb resources.
- JayDiff - A JSON diff utility written in Go.
- Terracognita - Reads from existing Cloud Providers (reverse Terraform) and generates your infrastructure as code on Terraform configuration.
- brotli - go bindings for Brotli algorithm.
- compress - Faster drop in replacements for gzip, zip, zlib, deflate.
- dgolzo - LZO bindings.
- dictzip - A reader and writer for files in the random access
dictzip
format. - fast-archiver - Alternative archiving tool with fast performance for huge numbers of small files.
- gbacomp - A Go library to (de)compress data compatible with GBA BIOS.
- go-lz4 - Port of LZ4 lossless compression algorithm to Go.
- go-lzss - Implementation of LZSS compression algorithm in Go.
- go-sevenzip - Package sevenzip implements access to 7-zip archives (wraps C interface of LZMA SDK).
- go-zip - A wrapper around C library libzip, providing ability to modify existing ZIP archives.
- lz4 - High performance, concurrent LZ4 implementation.
- lzma - compress/lzma package for Go.
- pgzip - Multicore gzip, compatible with the standard library.
- ppmd-go - Golang bindings for the LZMA SDK library. (Only binded PPMD)
- s2 - High throughput Snappy extension.
- snappy-go - Google's Snappy compression algorithm in Go.
- yenc - yenc decoder package.
- zappy - Package zappy implements the zappy block-based compression format. It aims for a combination of good speed and reasonable compression.
- zstd - Pure Go Zstandard compression/decompression.
- grpool - Lightweight Goroutine pool.
- pool - Go consumer goroutine pool for easy goroutine handling + time saving.
- tunny - A goroutine pool.
- worker - An easy and lightweight concurrent job framework.
- awsenv - a small binary that loads Amazon (AWS) environment variables for a profile
- confl - nginx config syntax, lenient, encode/decode, custom marshaling
- configor - Golang Configuration tool that support YAML, JSON, TOML, Shell Environment
- flagfile - Adds parsing and serialization support to the standard library flag package (adds a --flagfile option)
- gcfg - read INI-style configuration files into Go structs; supports user-defined types and subsections
- globalconf - Effortlessly persist to and read flag values from an ini config file
- goconf - a configuration file parser
- goconfig - Configuration based on struct introspection, supports environment vars, command line args, and more.
- hjson - Human JSON, a configuration file format for humans. Relaxed syntax, fewer mistakes, more comments.
- jsonconfig - a JSON configuration file parser with comments support
- koanf - Light weight, extensible library for reading config in Go applications. Built in support for JSON, TOML, YAML, env, command line.
- properties - Library for reading and writing properties files
- scribeconf - Facebook Scribe server configuration file parser
- toml:
- go-toml-config - TOML-based config for Go
- go-toml - Go library for the TOML language
- gp-config - Subset of TOML syntax with basic and reflection APIs
- toml-go - An easy-to-use Go parser for the Toml format
- toml - TOML parser for Go with reflection
- tom-toml - TOML parser for Go, support comments/formatter/apply.
- uConfig - an unopinionated, extendable and plugable configuration management. Supports YAML, TOML, JSON, Env vars, K8s DAPI, et al.
- viper - a complete configuration solution supporting YAML, TOML & JSON and integration with command line flags
- yaml:
code.soquee.net/env
— Load environment variables from.env
or similar files, or from anyio.Reader
and populate the local environment.
- ansi - Easily create ansi escape code strings and closures to format, color console output
- ansiterm - pkg to drive text-only consoles that respond to ANSI escape sequences
- cons - A simple package for building interactive console tools.
- gnureadline - GNU Readline bindings
- go-ansiout - Another ANSI escape code sequence tool for use with command-line applications.
- gockel - a Twitter client for text terminals
- gocui - Minimalist library aimed at creating Console User Interfaces
- gocurse - Go bindings for NCurses
- gocurses - NCurses wrapper
- go-ibgetkey - "hot key" type user input package for use in processing keystrokes in command-line applications.
- go.linenoise - Linenoise bindings (simple and easy readline with prompt, optional history, optional tab completion)
- goncurses - An ncurses library, including the form, menu and panel extensions
- gopass - Allows typing of passwords without echoing to screen
- go-pullbarz - Fancy "light bar" menus like in Lotus 123 from the DOS days. Dependent on go-ibgetkey and go-ansiout.
- go.sgr - Terminal/console colors and text decoration (bold,underlined,etc).
- go-stfl - a thin wrapper around STFL, an ncurses-based widget toolkit
- goterminal - A go library that lets you write and then re-write the text on terminal, to update progress. It works on Windows too!
- go-web-shell - Remote web shell, implements a net/http server.
- igo - A simple interactive Go interpreter built on exp/eval with some readline refinements
- oh - A Unix shell written in Go
- pty - obtain pseudo-terminal devices
- readline - A pure go implementation for GNU-Readline kind library
- termbox-go - A minimalist alternative to ncurses to build terminal-based user interfaces
- termios - Terminal support
- termon - Easy terminal-control-interface for Go.
- uilive - uilive is a go library for updating terminal output in realtime.
- uiprogress - A library to render progress bars in terminal applications.
- uitable - A library to improve readability in terminal apps using tabular data.
- yandex-weather-cli - Command line interface for Yandex weather service
- goveralls - Go integration for Coveralls.io continuous code coverage tracking system.
- overalls - Multi-Package go project coverprofile for tools like goveralls
- Skycoin - Skycoin is a next-generation cryptocurrency written in Go. Skycoin is not designed to add features to Bitcoin, but rather improves Bitcoin by increasing simplicity, security and stripping out everything non-essential.
- BLAKE2b - Go implementation of BLAKE2b hash function
- cryptogo - some useful cryptography-related functions, including paddings (PKCS7, X.923), PBE with random salt and IV
- cryptoPadding - Block padding schemes implemented in Go
- dkeyczar - Go port of Google'e Keyczar cryptography library
- dkrcrypt - Korean block ciphers: SEED and HIGHT
- dskipjack - Go implementation of the SKIPJACK encryption algorithm
- go-cs - concurrent ssh client.
- go-ed25519 - CGO bindings for Floodberry's ed25519-donna. Fast batch verification.
- go-hc128 - Go implementation of HC-128, an eSTREAM stream cipher
- go-jose - Go implementation of the JOSE standards
- go-minilock - Go implementation of the minilock file encryption system.
- GoSkein - Implementation of Skein hash and Threefisch crypto for Go
- keccak - A keccak (SHA-3) implementation
- ketama.go - libketama-style consistent hashing
- kindi - encryption command line tool
- openssl - openssl bindings for go
- otrcat - a general purpose command-line tool for communicating using the Off-The-Record protocol
- scrypt - Go implementation of Colin Percival's scrypt key derivation function
- simpleaes - AES encryption made easy
- siphash - SipHash: a fast short-input pseudorandom function
- SRP - SRP: Secure Remote Password - Implementation in go
- ssh.go - SSH2 Client library
- ssh-vault - encrypt/decrypt using ssh keys
- themis - Multi-platform high-level cryptographic library for protecting sensitive data: secure messaging with forward secrecy, secure data storage (AES256GCM); suits for building end-to-end encrypted applications
- tiger - Tiger cryptographic hashing algorithm
- whirlpool - whirlpool cryptographic hashing algorithm
- go-lioness - Lioness wide-block cipher using Chacha20 and Blake2b
- go-sphinxmixcrypto - Sphinx mix network cryptographic packet format operations
- automi - Compose process and integration flows on Go channels
- gostatsd - Statsd server and library.
- Heka - Real time data and log file processing engine.
- Kapacitor - Framework for processing, monitoring and alerting on timeseries data.
- pipe - Several functional programming supporting in golang (Map/Reduce/Filter)
- proto - Map/Reduce/Filter etc. for Go using channels as result streams.
- regommend - Recommendation engine.
- rrd - Bindings for rrdtool.
- XConv - Convert any value between types (base type, struct, array, slice, map, etc.)
- ratchet - A library for performing data pipeline / ETL tasks in Go.
- Glow - Glow is an easy-to-use distributed computation system, similar to Hadoop Map Reduce, Spark, Flink, Storm.
- Gleam - Fast, efficient, and scalable distributed map/reduce system, DAG execution, in memory or on disk, runs standalone or distributedly.
- collections - Several common data structures
- data-structures - A collection of data-structures (ArrayList, SortedList, Set, AVL Tree, Immutable AVL Tree, B+Tree, Ternary Search Trie, Hash Table (Separate Chaining), Linear Hash Table)
- ps - Persistent data structures
- Tideland golib - A collections library
- gohash - A simple linked-list hashtable that implements sets and maps
- go-maps - Go maps generalized to interfaces
- bimap - A simple bidirectional map implementation
- fs2/mmlist - A memory mapped list.
- GoArrayList - GoArrayList is a Go language substitute for the Java class ArrayList, with very nearly all features.
- goskiplist - A skip list implementation in Go.
- itreap - An immutable ordered list, internally a treap.
- ListDict - Python List and Dict for Go
- skip - A fast position-addressable ordered map and multimap.
- Skiplist - A fast indexable ordered multimap.
- skiplist - A skip list implementation. Highly customizable and easy to use.
- skiplist - Skiplist data structure ported from Redis's Sorted Sets.
- stackgo - A fast slice-based stack implementation.
- fifo_queue - Simple FIFO queue
- figo - A simple fifo queue with an optional thread-safe version.
- go.fifo - Simple auto-resizing thread-safe fifo queue.
- gopqueue - Priority queue at top of container/heap
- go-priority-queue - An easy to use heap implementation with a conventional priority queue interface.
- golibs/stack - A LIFO and ringbuffer package
- gringo - A minimalist queue implemented using a stripped-down lock-free ringbuffer
- heap - A general heap package without converting elements to
interface{}
and back. - queued - A simple network queue daemon
- queue - A queue manager on top of Redis
- graph - Library of basic graph algorithms
- graphs - Implementation of various tree, graph and network algorithms
- groph - A pure Go library of graphs and algorithms
- disjoint - Disjoint sets (union-find algorithm with path compression)
- golang-set - A full thread-safe and unsafe set implementation for Go.
- goset - A simple, thread safe Set implementation
- set - Set data structure for Go
- b - Package b implements B+trees with delayed page split/concat and O(1) enumeration. Easy production of source code for B+trees specialized for user defined key and value types is supported by a simple text replace.
- btree - Package btree implements persistent B-trees with fixed size keys, http://en.wikipedia.org/wiki/Btree
- btree - In-memory (not persistent) B-tree implementation, similar API to GoLLRB
- go-avltree - AVL tree (Adel'son-Vel'skii & Landis) with indexing added
- go-btree - Simple balance tree implementation
- go-darts - Double-ARray Trie System for Go
- GoLLRB - A Left-Leaning Red-Black (LLRB) implementation of 2-3 balanced binary search trees in Google Go
- go-merkle - Merkle-ized binary (search) trees with proofs.
- go-stree - A segment tree implementation for range queries on intervals
- go-radix, go-radix-immutable - Radix tree implementations.
- gtreap - Persistent treap implementation.
- rbtree - Yet another red-black tree implementation, with a C++ STL-like API
- rbtree - A high performance red-black tree with an API similar to C++ STL's for set, map, multiset, multimap.
- rtreego - an R-Tree library
- triego - Simple trie implementation for storing words
- prefixmap - Simple trie-based prefix-map for string-based keys
- aurora - Cross-platform Beanstalk queue server console.
- bigendian - binary parsing and printing
- deepcopy - Make deep copies of data structures
- dgobloom - A Bloom Filter implementation
- epochdate - Compact dates stored as days since the Unix epoch
- etree - Parse and generate XML easily
- excelize - Golang library for reading and writing Microsoft Excel™ (XLSX) files.
- fsm - Minimalistic state machine for use instead of booleans
- go-algs/ed - Generalized edit-distance implementation
- go-algs/maxflow - An energy minimization tool using max-flow algorithm.
- go-extractor - Go wrapper for GNU libextractor
- gocrud - Framework for working with arbitrary depth data structures.
- Gokogiri - A lightweight libxml wrapper library
- GoNetCDF - A wrapper for the NetCDF file format library
- goop - Dynamic object-oriented programming support for Go
- gopart- Type-agnostic partitioning for anything that can be indexed in Go.
- gotoc - A protocol buffer compiler written in Go
- govalid - Data validation library
- goxml - A thin wrapper around libxml2
- hyperloglog - An implementation of the HyperLogLog and HyperLogLog++ algorithms for estimating cardinality of sets using constant memory.
- itertools - Provides generic iterable generator function along with functionality similar to the itertools python package.
- jsonv - A JSON validator
- libgob - A low level library for generating gobs from other languages
- ofxgo - A library for querying OFX servers and/or parsing the responses (and example command-line client).
- Picugen - A general-purpose hash/checksum digest generator.
- tribool - Ternary (tree-valued) logic for Go
- Tuple - Tuple is a go type that will hold mixed types / values
- vcard - Reading and writing vcard file in go. Implementation of RFC 2425 (A MIME Content-Type for Directory Information) and RFC 2426 (vCard MIME Directory Profile).
- mxj - Marshal/Unmarshal XML doc from/to
map[string]interface{}
or JSON - xlsx - A library to help with extracting data from Microsoft Office Excel XLSX files.
- goxlsxwriter - Golang bindings for libxlsxwriter for writing XLSX (Excel) files
- simple-sstable - A simple, no-frills SSTable format and implementation in Go.
See also [[SQLDrivers page|SQLDrivers]].
- cockroachdb - A Scalable, Survivable, Strongly-Consistent SQL Database
- Hazelcast IMDG Go Client - The official Go client implementation for Hazelcast IMDG, the open source in-memory data grid.
- mgo - Rich MongoDB driver for Go
- rocks-stata - MongoDB Backup Utility
- Go-MySQL-Driver - A lightweight and fast MySQL-Driver for Go's database/sql package
- MyMySQL - MySQL Client API written entirely in Go.
- TiDB - MySQL compatible distributed database modeled after Google's F1 design.
- vitess - Scaling MySQL databases for the web
- mysqlsuperdump - Generate partial and filtered dumps of MySQL databases
- go-libpq - cgo-based Postgres driver for Go's database/sql package
- go-pgsql - A PostgreSQL client library for Go
- pgsql.go - PostgreSQL high-level client library wrapper
- pgx - Go PostgreSQL driver that is compatible with database/sql and has native interface for more performance and features
- pq - Pure Go PostgreSQL driver for database/sql
- yoke - Postgres high-availability cluster with auto-failover and automated cluster recovery
- kallax - PostgreSQL typesafe ORM
code.soquee.net/migrate
— A library for generating, applying, and listing PostgreSQL database migrations using a mechanism that's compatible with Rust's Diesel.
- ql - A pure Go embedded (S)QL database.
- godis - Simple client for Redis
- Go-Redis - Client and Connectors for Redis key-value store
- go-redis - Redis client built on the skeleton of gomemcache
- Redigo - Go client for Redis.
- redis - Redis client for Go
- GoRethink - RethinkDB Driver for Go
- gosqlite3 - Go Interface for SQLite3
- gosqlite (forked) - A fork of gosqlite
- gosqlite - a trivial SQLite binding for Go.
- go-sqlite - A database/sql driver and standalone sqlite3 interface
- go-sqlite-lite - A simple SQLite package for Go.
- mattn's go-sqlite3 - sqlite3 driver conforming to the built-in database/sql interface
- vertica-sql-go - A pure Go driver for Vertica database.
- beedb - beedb is an ORM for Go. It lets you map Go structs to tables in a database
- ent - An entity framework for Go
- FilterXorm - Build conditions for xorm project.
- go-modeldb - A simple wrapper around sql.DB for struct support.
- gorm - An ORM library for Go, aims for developer friendly
- gorp - SQL mapper for Go
- go-firestorm - Simple Go ORM for Cloud Firestore
- go-store - Data-store library for Go that provides a set of platform-independent interfaces to persist and retrieve key-value data.
- hood - Database agnostic ORM for Go. Supports Postgres and MySQL.
- lore - Simple and lightweight pseudo-ORM/pseudo-struct-mapping environment for Go.
- qbs - Query By Struct. Supports MySQL, PostgreSQL and SQLite3.
- sqlboiler - Database-first ORM via code generation.
- sqlgen - Go code generation for SQL interaction.
- structable - Struct-to-table database mapper.
- xorm - Simple and Powerful ORM for Go.
- reform - A better ORM for Go, based on non-empty interfaces and code generation.
- go-queryset - 100% type-safe ORM for Go with code generation and MySQL, PostgreSQL, Sqlite3, SQL Server support.
- bolt - Persistent key/value store inspired by LMDB.
- dbm - Package dbm (WIP) implements a simple database engine, a hybrid of a hierarchical and/or a key-value one.
- fs2/bptree - A memory mapped B+Tree with duplicate key support. Appropriate for large amounts of data (aka +100 GB). Supports both anonymous and file backed memory maps.
- Diskv - Home-grown, disk-backed key-value store
- etcd - Highly-available key value store for shared configuration and service discovery
- Olric Distributed and in-memory key/value database. It can be used both as an embedded Go library and as a language-independent service.
- gkvlite - Pure go, simple, ordered, atomic key-value persistence based on append-only file format.
- gocask - Key-value store inspired by Riak Bitcask. Can be used as pure go implementation of dbm and other kv-stores.
- goleveldb - Another implementation of LevelDB key/value in pure Go.
- kv - Yet another key/value persistent store. Atomic operations, two phase commit, automatic crash recovery, ...
- leveldb-go - This is an implementation of the LevelDB key/value database.
- levigo - levigo provides the ability to create and access LevelDB databases.
- persival - Programatic, persistent, pseudo key-value storage
- cayley - 100% Go graph database, inspired by Freebase and the Google Knowledge Graph.
- Dgraph - Fast, Distributed Graph DB with a GraphQL-like API.
- go-gremlin - A Go client for the Apache TinkerTop Graph analytics framework (Gremlin server).
- couchgo - The most feature complete CouchDB Adapter for Go. Modeled after couch.js.
- influxdb - Scalable datastore for metrics, events, and real-time analytics
- Kivik - Kivik provides a common Go and GopherJS client library for CouchDB, PouchDB, and similar databases.
- ledisdb - A high performance NoSQL like Redis.
- nodb - A pure Go embed Nosql database with kv, list, hash, zset, bitmap, set.
- tiedot - A NoSQL document database engine using JSON for documents and queries; it can be embedded into your program, or run a stand-alone server using HTTP for an API.
- cabinet - Kyoto Cabinet bindings for go
- camlistore - Personal distributed storage system for life.
- cdb.go - Create and read cdb ("constant database") files
- go-clickhouse - Connector to Yandex Clickhouse (column-oriented database)
- CodeSearch - Index and perform regex searches over large bodies of source code
- dbxml - A basic interface to Oracle Berkeley DB XML
- drive - A Google drive command line client
- Event Horizon - Toolkit for Command Query Responsibility Segregation and Event Sourcing (CQRS/ES)
- go-db-oracle - GO interface to Oracle DB
- gographite - statsd server in go (for feeding data to graphite)
- gokabinet - Go bindings for Kyoto Cabinet DBM implementation
- go-model - Robust & Easy to use struct mapper and utility methods for Go
- go-notify - GO bindings for the libnotify
- goprotodb - A binding to Berkeley DB storing records encoded as Protocol Buffers.
- go-rexster-client - Go client for the Rexster graph server (part of the TinkerPop suite of graph DB tools)
- goriak - Database driver for riak database (project homepage is now on bitbucket.org)
- goriakpbc - Riak driver using Riak's protobuf interface
- gotyrant - A Go wrapper for tokyo tyrant
- go-wikiparse - mediawiki dump parser for working with wikipedia data
- hdfs - go bindings for libhdfs
- JGDB - JGDB stands for Json Git Database
- mig - Simple SQL-based database migrations
- mongofixtures - A Go quick and dirty utility for cleaning MongoDB collections and loading fixtures into them.
- Neo4j-GO - Neo4j REST Client in Go
- neoism - Neo4j graph database client, including Cypher and Transactions support.
- null - Package for handling null values in SQL
- Optimus Cache Prime - Smart cache preloader for websites with XML sitemaps.
- piladb - Lightweight RESTful database engine based on stack data structures.
- pravasan - Simple Migration Tool (like rake db:migrate with more flexibility)
- riako - High level utility methods for interacting with Riak databases
- sqlbuilder - SQL query builder with row mapping
- sqlf - Create parameterized SQL statements in Go, sprintf style
- squirrel - Fluent SQL generation for Go
- Sublevel - Separate sections of the same LevelDB
- Weed File System - fast distributed key-file store
- whisper-go - library for working with whisper databases
- remapper - library to convert/map data from one type to another
- xo - CLI to generate idiomatic Go code for databases
- go-batcher - Simply create and use batch handler in Go
- now - Now is a time toolkit for golang.
- date - A package for working with dates.
- date - For dates, date ranges, time spans, periods, and time-of-day.
- gostrftime - strftime(3) like formatting for time.Time
- goment - Go time library inspired by Moment.js
- tai64 - tai64 and tai64n parsing and formatting
- tuesday - A Strftime implementation that's compatible with Ruby's
Time.strftime
- Tideland golib - Timex extensions
- hijri - A small helper library to convert a Hijri date to a Gregorian date according to Ummul Qura calendar.
- goyaccfmt - Auto reformat Goyacc source files.
- cwrap - Go wrapper (binding) generator for C libraries.
- demand - Download, build, cache and run a Go app easily.
- glib - Bindings for GLib type system
- go-callvis - Visualize call graph of your Go program using dot format.
- gocog - A code generator that can generate code using any language
- godepgraph - Create a dependency graph for a go package
- godev - Recompiles and runs your Go code on source change. Also watches all your imports for changes.
- godiff - diff file comparison tool with colour html output
- gonew - A tool to create new Go projects
- go-play - A HTML5 web interface for experimenting with Go code. Like http://golang.org/doc/play but runs on your computer
- gore - A Go REPL. Featured with line editing, code completion, and more
- gorun - Enables Go source files to be used as scripts.
- go-spew - Implements a deep pretty printer for Go data structures to aid in debugging
- goven - Easily copy code from another project into yours
- gowatcher - Reload a specified go program automatically by monitoring a directory.
- GoWatch - GoWatch watches your dev folder for modified files, and if a file changes it restarts the process.
- goweb - Literate programming tools for Go based on CWEB by Donald Knuth and Silvio Levy.
- hopwatch - simple debugger for Go
- hsandbox - Tool for quick experimentation with Go snippets
- Inject - Library for dependency injection in Golang (from Facebook)
- liccor - A tool for updating license headers in Go source files
- liteide - An go auto build tools and qt-based ide for Go
- Livedev - Livedev is a development proxy server that enables live code reloading.
- Martian - HTTP proxy designed for use in E2E testing.
- nvm-windows - Node.js version manager for Windows
- prettybenchcmp - Store and compare benchmarks history locally.
- rerun - Rerun watches your binary and all its dependencies so it can rebuild and relaunch when the source changes.
- syntaxhighlighter - language-independent code syntax highlighting library
- toggle - A feature toggle library with built in support for environment variable backed toggling. pluggable backing engine support.
- trace - A simple debug tracing
- sling - Network traffic simulator and test automation tool to send file requests through the HTTP or TCP protocol, control rate frequency, number of concurrent connections, delays, timeouts, and collect the response time statistics, mean, and percentiles.
- egotags - ETags generator
- tago1 - etags generator for go that builds with go 1
- tago - Emacs TAGS generator for Go source
- celeriac - A library for adding support for interacting and monitoring Celery workers, tasks and events in Go
- donut - A library for building clustered services in Go
- libchan - Go-like channels over the network
- locker - A distributed lock service built on top of etcd.
- dlock - A native Go distributed lock manager (client and server) using gRPC.
- mangos - An implementation of the Scalable Protocols based on nanomsg
- redsync - Redis-based distributed mutual exclusion lock implementation
- Skynet - Skynet is distributed mesh of processes designed for highly scalable API type service provision.
- Tideland golib - Includes a map/reduce library
- examplgen - Insert code from .go files to documents (examples to project's readme, for instance).
- godocdown - Format package documentation (godoc) as GitHub friendly Markdown
- GoDoc.org - GoDoc.org generates documentation on the fly from source on Bitbucket, Github, Google Project Hosting and Launchpad.
- golangdoc - Godoc for Golang, support translate.
- Mango - Automatically generate unix man pages from Go sources
- redoc - Commands documentation for Redis
- sphinxcontrib-golangdomain - Sphinx domain for Go
- test2doc - Generate documentation for your go units from your unit tests.
- A - A graphical text and binary editor based on Acme
- Conception - Conception is an experimental research project, meant to become a modern IDE/Language package. demo video
- de - A modal graphical editor with Acme and vi features
- Go-bbpackage - BBEdit package for Go development
- goclipse - An Eclipse-based IDE for Go.
- Go conTEXT - Highlighter plugin
- godev - Web-based IDE for the Go language
- godit - A microemacs-like text editor written in Go.
- gofinder - (code) search tool for acme
- go-gedit - Google Go language plugin for gedit
- Gide - Go IDE built in the go-native GoGi GUI
- golab - go local application builder - a web-based Go ide
- Google Go for Idea - Google Go language plugin for Intellij IDEA
- micro - A modern and intuitive terminal-based text editor.
- T - An Acme/Sam like text editor
- tabby - Source code editor
- ViGo - A vim-like text editor.
- Wide - A Web-based IDE for Teams using Golang.
- Hectane - Lightweight SMTP client including a built-in mail queue backed by on-disk storage.
- gmail - Simple library for sending emails from a Gmail account, for people not interested in dealing with protocol details.
- go-mail - Email utilities including RFC822 messages and Google Mail defaults.
- Gomail - A simple and efficient package to send emails.
- go-ses - Amazon AWS Simple Email Service (SES) API client
- Inbucket - Inbucket is an email testing service; it will accept messages for any email address and make them available to view via a web interface.
- mail.go - Parse email messages
- MailHog - Email testing service, inspired by MailCatcher.
- MailSlurper - A handy SMTP mail server useful for local and team application development. Slurp mail into oblivion!
- chasquid - SMTP server written in Go.
- hierr - Nesting errors in hierarchy.
- errgo - Error tracing and annotation.
- errors - The juju/errors package provides an easy way to annotate errors without losing the original error context, and get a stack trace back out of the error for the locations that were recorded.
- errors - errors augments and error with a file and line number.
- goerr - Allows to make a separate(modular) and reusable error handlers. Exception-like panic() recover() mechanism using Return(error) and catching err := OR1(..)
- panicparse - Parse panics with style.
- Space Monkey errors - Go's missing errors library - stack capture, error hierarchies, error tags
- Tideland golib - Detailed error values
code.soquee.net/problem
— Package problem implements errors for HTTP APIs similar to the ones described by RFC7807.
- base58 - Human input-friendly base58 encoding
- bencode-go - Encoding and decoding the bencode format used by the BitTorrent peer-to-peer file sharing protocol
- bsonrpc - BSON codec for net/rpc
- chardet - Charset detection library ported from ICU
- charmap - Character encodings in Go
- codec-msgpack-binc High Performance and Feature-Rich Idiomatic Go Library providing encode/decode support for multiple binary serialization formats: msgpack
- colfer - high-performance binary codec
- gobson - BSON (de)serializer
- go-charset - Conversion between character sets. Native Go.
- go.enmime - MIME email parser library for Go (native)
- go-msgpack - Comprehensive MsgPack library for Go, with pack/unpack and net/rpc codec support (DEPRECATED in favor of codec )
- gopack - Bit-packing for Go
- go-simplejson - a Go package to interact with arbitrary JSON
- go-wire - Binary and JSON codec for structures and more
- go-xdr - Pure Go implementation of the data representation portion of the External Data Representation (XDR) standard protocol as specified in RFC 4506 (obsoletes RFC 1832 and RFC 1014).
- iconv-go - iconv wrapper with Reader and Writer
- magicmime -- Mime-type detection with Go bindings for libmagic
- Mahonia - Character-set conversion library in Go
- mimemagic - Detect mime-types automatically based on file contents with no external dependencies
- mimemagic - A pure-Go MIME sniffing library/tool based on the FreeDesktop.org spec
- msgpack - Msgpack format implementation for Go
- msgpack-json - Command-line utilities to convert between msgpack and json
- nnz - String and Int primitives that serialize to JSON and SQL null
- storable - Write perl storable data
- TNetstring - tnetstrings (tagged Netstrings)
- afero - A File Sytem abstraction system for Go
- go.fs - A virtual file system abstraction layer.
- poller - Package poller is a file-descriptor multiplexer. It allows concurent Read and Write operations from and to multiple file-descriptors.
- vfsgen - Generates a vfsdata.go file that statically implements the given virtual filesystem.
- Bampf - Arcade style game based on the Vu 3D engine.
- bloxorz - Solver for bloxorz basic levels
- ChessBuddy - Play chess with Go, HTML5, WebSockets and random strangers!
- Fergulator - An NES emulator, using SDL and OpenGL
- FlappyBird - A simple flappy bird clone written in golang.
- godoku - Go Sudoku Solver - example of "share by communicating"
- Gongo - A program written in Go that plays Go
- gospeccy - A ZX Spectrum 48k Emulator
- Ludo Game - Ludo Board game powered by Go on Appengine
- pinkman - command line based chess interface to UCI compatible chess engines
- Pong - A simple Pong clone written in golang
- pong-command - Joke command,ping-like pong.
- Steven - A Minecraft client in Go
- ukodus - Sudoku solver in Go
- WolfenGo - A Wolfenstein3D clone in Go, using OpenGL 2.1
- geojson - Go package to quick and easy create json data in geojson format. description
- go-geom - Efficient Open Geo Consortium-style geometries with native Go GeoJSON and WKB encoding and decoding (work-in-progress)
- go.geo - Geometry/geography library targeted at online mapping (deprecated by author in favor of his new gis library
orb
.) - go.geojson - Marshalling and Unmarshalling of GeoJSON objects
- gogeos - Go library for spatial data operations and geometric algorithms
- go-proj-4 - An interface to the Cartographic Projections Library PROJ.4
- go-kml - Google Earth KML generation
- go-polyline - Google Maps polyline encoding and decoding
- orb - 2d geometry manipulation (length, area, polygon contains, etc.) with geojson, wkb, mvt support
- osm - General purpose library for reading, writing and working with OpenStreetMap data
- UTM - Bidirectional UTM-WGS84 converter
- gdal - Provides a go wrapper for GDAL
- llgo - LLVM-based Go compiler, written in Go
- AnsiGo - Simple ANSi to PNG converter written in pure Go
- Arclight - Arclight is a tool for rendering images
- bild - A collection of image processing algorithms in pure Go
- bimg - Small Go library for fast image resize and transformation using libvips
- blend - Image processing library and rendering toolkit for Go.
- bpg - BPG decoder for Go.
- chart - Library to generate common chart (pie, bar, strip, scatter, histogram) in different output formats.
- draw2d - This package provide an API to draw 2d geometrical form on images. This library is largely inspired by postscript, cairo, HTML5 canvas.
- ebiten - A cross platform open-source game library with which you can develop 2D games with simple API for multi platforms. Cgo/c compiler setup not needed.
- egl - egl bindings
- es2 - es2 bindings
- fourcc - Go implementation of FOURCC (four character code) (4CC) identifiers for a video codecs, compression formats, colors, and pixel format used in media files.
- freetype-go - a Go implementation of FreeType
- gltf - library for marshaling and unmarshaling glTF
- glfw 3 - Go bindings for GLFW 3 library
- glfw - bindings to the multi-platform library for opening a window, creating an OpenGL context and managing input
- glh - OpenGL helper functions to manage text, textures, framebuffers and more
- gl - OpenGL bindings using glew
- glu - bindings to the OpenGL Utility Library
- gmask - Go adaptation of the Cmask utility for Csound
- goalsa - Go bindings for ALSA capture and playback
- go-cairo - Go wrapper for the cairo graphics library
- gocl - Go OpenCL (gocl) binding, support OpenCL 1.1/1.2/2.0 on Mac/Linux/Windows/Android
- go-csnd6 - Go binding to the Csound6 API
- go-csperfthread - Go binding to the CsoundPerformanceThread helper class of the Csound6 API
- goexif - Retrieve EXIF metadata from image files
- goflac - Go bindings for decoding and encoding FLAC audio with libFLAC
- go-gd - Go bingings for GD
- GoGL - OpenGL binding generator
- go-gnuplot - go bindings for Gnuplot
- go-gtk3 - gtk3 bindings for go
- go-heatmap - A toolkit for making heatmaps
- GoHM - H.265/HEVC HM Video Codec in Go
- goHorde - Go Bindings for the Horde3d Rendering engine.
- GoMacDraw - A mac implementation of go.wde
- go-openal - Experimental OpenAL bindings for Go
- go-opencl - A go wrapper to the OpenCL heterogeneous parallel programming library
- go-opencv - Go bindings for OpenCV / 2.x API in gocv / 1.x API in opencv
- Go-OpenGL - Go bindings for OpenGL
- Goop - Audio synthesizer engine
- goray - Raytracer written in Go, based on Yafaray
- gosc - Pure Go OSC (Open Sound Control) library
- go-taglib - Go wrapper for TagLib, an audio meta-data parser
- go-tmx - A Go library that reads Tiled's TMX files
- GoVisa - H265/HEVC Bitstream Analyzer in Go
- go-vlc - Go bindings for libVLC
- go.wde - A windowing/drawing/event interface
- goxscr - Go rewrites of xscreensaver ports
- gst - Go bindings for GStreamer
- gumble - Client library for the Mumble VoIP protocol
- hgui - Gui toolkit based on http and gtk-webkit.
- imaginary - Simple and fast HTTP microservice for image resizing and manipulation
- imaging - Package imaging provides basic image manipulation functions (resize, rotate, flip, crop, etc.) as well as simplified image loading and saving.
- imgproxy - Fast and secure standalone server for resizing and converting remote images
- merlin - Automatic video encoder
- netpbm - Read and write Netpbm images from Go programs
- opencv - Go bindings for OpenCV
- osmesa - Go bindings for osmesa.
- phono - DSP pipeline.
- Plotinum - An API for creating plots
- portaudio - A Go binding to PortAudio
- pulsego - Go binding for PulseAudio
- pulse-simple - Go bindings for PulseAudio's Simple API, for easy audio capture and playback.
- rasterx - SVG-standard rendering, rasterization library.
- resize - Image resizing with different interpolations.
- RiGO - RenderMan Interface implementation in Go.
- smartcrop - Content aware image cropping
- starfish - A simple Go graphics and user input library, built on SDL
- stl - library for reading, writing, and manipulating Stereolithography (.stl) files used in 3D printing
- svgo - a library for creating and outputting SVG
- tag - a library for reading tag metadata and creating metadata-invariant checksums for audio files: FLAC, all IDv3 variants, and MP4 (ACC, ALAC)
- tga - TARGA image format encoding/decoding library
- tiff - Rich TIFF/BigTIFF/GeoTIFF decoder/encoder for Go.
- twilio-go - Go client for the Twilio API.
- videoinput - Go bindings for VideoInput (Windows).
- vu - Virtual Universe. A skeleton 3D engine.
- vulkan - Golang Bindings for Vulkan API.
- webp - WebP decoder and encoder for Go.
- wg - Web Graphics, display real time go graphics in browser, with user input.
- window - Optimized moving window for real-time data
- libvlc-go - Go bindings for libVLC 2.X/3.X/4.X used by the VLC media player
- go-fltk - FLTK2 GUI toolkit bindings for Go
- go-gtk - Bindings for GTK
- go-qt5 - QT5 bindings for go
- GoGi - Fully native cross-platform GUI toolkit
- gothic - Tcl/Tk Go bindings
- gotk3 - Go bindings for GTK3, requires GTK version 3.8
- go.uik - A UI kit for Go, in Go. (project is closed)
- go-webkit2 - Go bindings for the WebKitGTK+ v2 API (w/headless browser & JavaScript support)
- Gowut - Gowut (Go Web UI Toolkit) is a full-featured, easy to use, platform independent Web UI Toolkit written in pure Go, no platform dependent native code is linked or called.
- GXUI - A Go cross platform UI library.
- iup - Bindings for IUP
- lorca - A small library for building cross-platform HTML5 GUI apps in Go, uses Chrome/Chromium as a UI layer.
- mdtwm - Tiling window manager for X
- qml - QML support for the Go language
- ui - Platform-native GUI library for Go
- webview - Tiny cross-platform web UI library. Uses WebKit (Gtk/Cocoa) and MSHTML (Windows)
- wingo - A fully-featured window manager written in Go.
- Winhello - An example Windows GUI hello world application
- wxGo - Go Wrapper for the wxWidgets GUI
- xgb - A fork of the x-go-binding featuring support for thread safety and all X extensions.
- xgbutil - A utility library to make use of the X Go Binding easier. (Implements EWMH and ICCCM specs, key binding support, etc.)
- x-go-binding - bindings for the X windowing system
- go.hid - Provides communication with USB Human Interface Devices.
- gortlsdr - A librtlsdr wrapper, which turns certain USB DVB-T dongles into a low-cost, general purpose software-defined radio receiver.
- hwio - Hardware I/O library for SoC boards including BeagleBone Black and Raspberry Pi.
- stressdisk - Stress test your disks / memory cards / USB sticks before trusting your valuable data to them
- gobot - Golang framework for robotics, drones, and the Internet of Things (IoT).
- alpinocorpus-go - A reader and a writer for Alpino corpora.
- go-aspell - GNU Aspell spell checking library bindings for Go.
- go-language - A simple language detector using letter frequency data.
- go-ngram - An n-gram is a contiguous sequence of n items from a given sequence of text or speech.
- go-tokenizer - A Text Tokenizer library for Golang
- go.stringmetrics - String distance metrics implemented in Go
- goling - String Similarity(Cosine Similarity, Levenshtein Distance), Spell Check, Segmentation
- go-l10n - localization for humans
- inflect - Word inflection library (similar to Ruby ActiveSupport::Inflector). Singularize(), Pluralize(), Underscore() etc.
- libtextcat - A Go wrapper for libtextcat.
- nlp - Go Natural Language Processing library supporting LSA (Latent Semantic Analysis).
- sego - Chinese language segmenter.
- snowball - Snowball stemmers for multiple languages
- textcat - N-gram based text categorization, with support for utf-8 and raw text
- colog - CoLog is a prefix-based leveled execution log for Go
- cue - Fast and flexible contextual logger. Supports output to file, syslog, structured syslog, stdout/stderr, socket, Loggly, Honeybadger, Opbeat, Rollbar, and Sentry.
- epazote - Automated Microservices Supervisor.
- factorlog - Really fast, featureful logging infrastructure (supports colors, verbosity, and many formats)
- vlog - Leveled log on std log for Go
- glog - Leveled execution logs for Go
- go-logging - Supports different logging backends like syslog, file and memory. Multiple backends can be utilized with different log levels per backend and logger.
- gomol - A multi-output logging library designed for outputs that support additional metadata with log messages.
- gosrvmon - Self-hosted uptime monitoring system.
- graylog-golang - graylog-golang is a full implementation for sending messages in GELF (Graylog Extended Log Format) from Google Go (Golang) to Graylog
- haminer - Library and program to parse and forward HAProxy logs
- jWalterWeatherman - Seamless terminal printing and file logging that’s as easy to use as fmt.Println
- immortal - A *nix cross-platform (OS agnostic) supervisor
- log4go - Go logging package akin to log4j
- logger - Go logging with buffered output and multiple writers
- logrus - Structured, pluggable logging for Go with built-in hooks for third-party loggers: Airbrake, Papertrail, Loggly, Sentry...
- MailJet Live Event Dashboard - API monitoring in real time.
- monkit - A flexible process data collection, metrics, monitoring, instrumentation, and tracing library for Go
- Prometheus - Monitoring system and time-series database.
- rfw - Rotating file writer - a 'logrotate'-aware file output for use with loggers
- sd - Writes to the systemd journal, supports user defined systemd journal fields
- seelog - Flexible dispatching, filtering, and formatting
- snap - Telemetry framework
- spacelog - Hierarchical, leveled, and structured logging library for Go
- statsgod - A rewrite of StatsD in Go.
- syslog - With this package you can create your own syslog server with your own handlers for different kind of syslog messages
- Tideland golib - Flexible logging
- timber - Configurable Logger for Go
- ul - Provides macOS Sierra/OSX Unified Loggging functionality via cgo
- bayesian - A naive bayes classifier.
- ctw - Context Tree Weighting and Rissanen-Langdon Arithmetic Coding
- evo - a framework for implementing evolutionary algorithms in Go.
- go-algs/maxflow Maxflow (graph-cuts) energy minimization library.
- go-galib - Genetic algorithms.
- golinear - Linear SVM and logistic regression.
- go_ml - Linear Regression, Logistic Regression, Neural Networks, Collaborative Filtering, Gaussian Multivariate Distribution.
- gorgonia - Neural network primitives library (like Theano or Tensorflow but for Go)
- go-porterstemmer - An efficient native Go clean room implementation of the Porter Stemming algorithm.
- go-pr - Gaussian classifier.
- ntm - Neural Turing Machines implementation
- paicehusk - Go implementation of the Paice/Husk Stemmer
- go-mind - A neural network library built in Go
- Anna - Artificial Neural Network Aspiration, aims to be self-learning and self-improving software.
- Dialex - Dialex is a smart pipe that unscrambles text and makes it machine-readable.
- tfgo - Tensorflow + Go, the gopher way.
- bayesian - Naive Bayesian Classification for Go
- blas - Go implementation of BLAS (Basic Linear Algebra Subprograms)
- cartconvert - cartography functions for the Go programming language
- clp - Go bindings for the COIN-OR Linear Programming (CLP) library
- Cvx - Convex optimization package, port of CVXOPT python package
- dice - Dice rolling library
- evaler - A simple floating point arithmetic expression evaluator
- fixed - A fixed point (Q32.32 format) math library
- geom - 2d geometry
- gini - SAT Solver/Boolean Logic Tools
- gochipmunk - Go bindings to the Chipmunk Physics library
- gocomplex - a complex number library
- godec - multi-precision decimal arithmetic
- gofd - concurrent finite domain constraint solver.
- go-fftw - Go bindings for FFTW - The Fastest Fourier Transform in the West
- go-fn - Special functions that would not fit in "math" pkg
- gographviz - Graphviz DOT language parser for Go
- go-gt - Graph theory algorithms
- go-humanize - Formatting numbers for humans.
- golibs/xmath - a collection of math functions (mostly mean algorithms)
- go-lm - Linear models in Go. Provides WLS and regression with t residuals via a cgo -> BLAS/LAPACK interface.
- go.mahalanobis - Naive implementation of the Mahalanobis distance using go.matrix
- gomat - lightweight FAST matrix and vector math
- go_matrix_cuda - GPU-Accelerated Linear Algebra Libraries based in CUDA
- go.matrix - a linear algebra package
- gonum - Scientific packages (linear algebra, BLAS, LAPACK, differentiation, plots, linear programming, statistics, ...)
- go-symexpr - Symbolic math as an AST with derivatives, simplification, and non-linear regression
- gsl - GNU Scientific Library bindings
- interval - Package interval handles sets of ordered values laying between two, possibly infinite, bounds.
- mathutil - Package mathutil provides utilities supplementing the standard 'math' and 'rand' packages.
- mt19937_64 - Mersenne Twister int64 random source
- permutation - Package permutation generates permutations of the indices of a slice
- polyclip.go - Go implementation of algorithm for Boolean operations on 2D polygons
- primes - Simple functionality for working with prime numbers.
- prime - Go version of Segmented Sieve and non Segmented sieve to produce prime numbers
- primegen.go - Sieve of Atkin prime number generator
- pso-go - A library of PSO (Particle Swarm Optimization) for Go.
- rand - 64-bit version of the math/rand package with Mersenne twister support.
- roger - A Go client for the RServer, allowing you to invoke R programs from Go.
- sparse - Go Sparse matrix formats for linear algebra supporting scientific and machine learning applications, compatible with gonum matrix libraries.
- stats - A statistics package with common functions missing from the Golang standard library.
- statistics - GNU GSL Statistics (GPLv3)
- Tideland golib - Numerics package for statistcal analysis
- Units - Implements types, units, converter functions and some mathematics for some common physical types. lib
- vector - A small vector lib.
- humanize - formats large numbers into human readable small numbers
- gokit - The Go Kit microservice framework (and author interview).
- go-micro - Go Micro is a microservices library which provides the fundamental building blocks for writing fault tolerant distributed systems at scale.
- kite - RPC server and client framework.
- car_registration - API wrapper for worldwide car registration data
- atexit - Simple atexit library
- bíogo - Basic bioinformatics functions for the Go language.
- Breaker - Breaker enables graceful degraded mode operations by means of wrapping unreliable interservice interface points with circuit breaker primitives.
- btcrpcclient - A Websocket-enabled Bitcoin JSON-RPC client.
- cast - Safe and easy casting from one type to another in Go
- CGRates - Rating system designed to be used in telecom carriers world
- cpu - A Go package that reports processor topology
- cron - A library for running jobs (funcs) on a cron-formatted schedule
- daemonigo - A simple library to daemonize Go applications.
- dbus-go - D-Bus Go library
- desktop - Open file/uri with default application (cross platform)
- devboard - Kanban board application based on Simple-Khanban
- dioder-api - An API to IKEA dioder LED-strips
- doublejump - A revamped Google's jump consistent hash
- dump - An utility that dumps Go variables, similar to PHP's var_dump
- elPrep - A high-performance tool for preparing sequence alignment/map files in DNA sequencing pipelines
- env - Easily pull environment variables with defaults
- epub - Bindings for libepub to read epub content.
- EventBus - Lightweight event bus with async compatibility for Go .
- faker - Generate fake data, names, text, addresses, etc.
- fsnotify - File system notifications for Go
- functional - Functional programming library including a lazy list implementation and some of the most usual functions.
- GCSE - Go code search engine. source
- generate - runs go generate recursively on a specified path or environment variable and can filter by regex.
- go-amiando - Wrapper for the Amiando event management API
- go-bit - An efficient and comprehensive bitset implementation with utility bit functions.
- go-bitops - common bit operations for 32/64 bit integer
- go-business-creditcard - Validate/generate credit card checksums/names.
- gochem - A computational chemistry/biochemistry library.
- gocsv - Library for CSV parsing and emitting
- go.dbus - Native Go library for D-Bus
- go-ean - A minimal utility library for validating EAN-8 and EAN-13 and calculating checksums.
- go-eco - Functions for use in ecology
- go-erx - Extended error reporting library
- go-eventsocket - An event socket client/server library for the FreeSWITCH telephony platform.
- GoFakeIt - Fake Data Generator. 65+ different variations and examples for each
- go-fann - Go bindings for FANN, library for artificial neural networks
- GoFlow - Flow-based and dataflow programming library for Go
- goga - A genetic algorithm framework
- gogobject - GObject-introspection based bindings generator
- go-idn - a project to bring IDN support to Go, feature compatible with libidn
- GoLCS - Solve Longest Common Sequence problem in go
- golibs/as - Converting data types
- golife - Implementation of Game of Life for command line
- go-magic - A Go wrapper for libmagic
- go-magic - Simple interface to libmagic for Go Programming Language
- go-metrics - Go port of Coda Hale's Metrics library
- gommap - gommap enables Go programs to directly work with memory mapped files and devices in a very efficient way
- gomusicbrainz - MusicBrainz WS2 client library
- goneuro - Go driver for NeuroSky devices.
- goNI488 - A Go wrapper around National Instruments NI488.2 General Purpose Interface Bus (GPIB) driver.
- go-osx-plist - CoreFoundation Property List support for Go
- go-papi - Go interface to the PAPI performance API
- go.pcsclite - Go wrapper for pcsc-lite
- Go-PhysicsFS - Go bindings for the PhysicsFS archive-access abstraction library.
- go.pipeline - Library that emulates Unix pipelines
- go-pkg-mpd - A library to access the MPD music daemon
- go-pkg-xmlx - Extension to the standard Go XML package. Maintains a node tree that allows forward/backwards browser and exposes some simpel single/multi-node search functions
- goplan9 - libraries for interacting with Plan 9
- goPromise - Scheme-like delayed evaluation for Go
- go-qrand - Go client for quantum random bit generator service at random.irb.hr
- goraphing - A tool to generate a simple graph data structures from JSON data files
- go-selenium - Selenium WebDriver client for Go
- go-semvar - Semantic versions (see http:/semver.org)
- go-serial - Go binding to libserialport for serial port functionality (cgo).
- goST - A steam properties (steam table) library written for Go. This was designed as a native go equivalent to XSteam.
- go-taskstats - Go interface for Linux taskstats
- gotenv - Loads environment variables from
.env
file - Gotgo - A Go preprocessor that provides an implementation of generics
- go-translate - Google Language Translate library
- go-uuid - Universal Unique IDentifier generator and parser
- gouuid - Pure Go UUID v3, 4 and 5 generator compatible with RFC4122
- go-villa - Some miscellaneous wrapper and small algorithms.(wrappers to slices, priority queues, path related apis, a string set type)
- Hranoprovod - Command-line calorie tracking
- lineup - A minimalistic message queue server
- mitigation - Package mitigation provides the possibility to prevent damage caused by bugs or exploits.
- nma.go - A NotifyMyAndroid client for go.
- notify - File system event notification library with API similar to os/signal.
- pargo - A library for parallel programming in Go.
- passwd - A parser for the /etc/passwd file
- pool - A generic worker pool
- procfile - A Procfile parser
- Prometheus Instrumentation/Metrics Client - This is a whitebox instrumentation framework for servers written in Go. It exposes programmatically-generated metrics automatically for use in the Prometheus time series collection and post-processing environment.
- randat - Devel tool for generating random bytestrings and encoding files in code-friendly forms
- recycler - A more flexible object recycling system than sync.Pool. Provides constructors and destructors for the objects as well as control over the length the free.
- replaykit - A library for replaying time series data.
- serial - Serial ports API (pure Go)
- sio - Package sio lets you access old serial junk. It's a go-gettable fork and modification of dustin's rs232 package.
- stats - Monitors Go MemStats + System stats such as Memory, Swap and CPU and sends via UDP anywhere you want for logging etc...
- symutils - Various tools and libraries to handle symbolic links
- toktok - Creates and resolves unique, typo & error-resilient, human-readable tokens
- twitterfetcher - A tool to make Twitter API requests using the Application-only authentication
- udis86 - Go bindings for libudis86
- ugo - underscore.js like toolbox for Go
- Vboxgo - user-like access to VirtualBox VMs from Go.
- vk - unofficial vk.com API wrapper (vk.com — russian social network)
- WUID - An extremely fast unique number generator, 10-135 times faster than UUID.
- Wukong - A highly extensible full-text search engine written in Go.
- xplor - Files tree browser for p9p acme
- yubigo - Yubikey OTP validation and auhtentication API client.
- go-wkhtmltopdf - wkhtmltopdf Go bindings and high level interface for HTML to PDF conversion.
- gmask - Go adaptation of the Cmask utility for Csound
- go-csnd6 - Go binding to the Csound6 API
- go-csperfthread - Go binding to the CsoundPerformanceThread helper class of the Csound6 API
- go-libshout - Go bindings for libshout
- gompd - A client interface for the MPD (Music Player Daemon)
- launchpad - A Go client for Novation Launchpad
- portmidi - Go bindings for libportmidi
- bitz - BitMessage client node and library
- dingo - A DNS client in Go that supports Google DNS over HTTPS
- dns - A DNS library in Go
- dnsimple - an interface to the DNSimple API
- dyndnscd - a configurable dyndns client
- GeoDNS - geo-aware authoritative DNS server
- grong - Small authoritative DNS name server
- lib/dns - The DNS library for client or server with support UDP, TCP, and DNS over HTTPS
- mdns - Multicast DNS library for Go
- hostsfile - /etc/hostsfile reverse lookup IP => names
- dnss - DNS secure proxy, supports DNS over HTTPS and GRPC
- domainerator - Command line tool to combine wordlist and suffixes/TLDs into domain names and check if they are registered or not.
- dns - client and server implementations in Go
- rescached - DNS resolver cache daemon
- ftp4go - An FTP client for Go, started as a port of the standard Python FTP client library
- ftp - Package ftp provides a minimal FTP client as defined in RFC 959
- ftps - An implementation of the FTPS protocol
- goftp - A FTP client library
- apiproxy - proxy for HTTP/REST APIs with configurable cache timeouts
- boom - HTTP(s) benchmarking tool, Apache Benchmark replacement
- eventsource - Server-sent events for net/http server.
- fasthttp - Fast HTTP package for Go
- gbench - HTTP(s) Load Testing And Benchmarking Tool inspired by Apache Benchmark and Siege.
- gobench - HTTP/HTTPS load test and benchmark tool
- go-curl - libcurl bingding that supports go func callbacks
- goproxy - a programmable HTTP proxy.
- gostax - A Streaming API for XML (StAX) in go
- handlers - Collection of useful HTTP middlewares.
- HTTPLab - HTTPLabs let you inspect HTTP requests and forge responses.
- httpmock - Easy mocking of HTTP responses from external resources
- stress - Replacement of ApacheBench(ab), support for transactional requests, support for command line and package references to HTTP stress testing tool.
- sling - A Go HTTP client library for creating and sending API requests.
- httptail - tools push stdout/stderr to http chunked
- go-imap - An IMAP library for clients and servers.
- go-imap - IMAP client library
- go-imap - Implementation of IMAP4rev1 client, as described in RFC 3501
- hanu - Framework for writing Slack bots
- gobir - Extensible IRC bot with channel administration, seen support, and go documentation querying
- goexmpp - XMPP client implementation
- goirc - event-based stateful IRC client framework
- go-irc - Simple IRC client library
- gorobot - a modular IRC bot
- go-xmpp - XMPP client library
- ircflu - IRC bot with support for commands, scripting and web-hooks
- irc.go - Go IRC bot framework
- sirius - [link is broken] A fast and ultra-lightweight chat server written in Go
- xmpp-client - an XMPP client with OTR (off-the-record) support
mellium.im/xmpp
— a low-level XMPP client and server library focusing on good documentation and a clean, usable API
- go-nntp - An NNTP client and server library for go
- gogoprotobuf - another Go implementation of Protocol Buffers, but with extensions and code generation plugins.
- golang_protobuf_extensions - Protocol Buffer extensions to support streaming message encoding and decoding.
- goprotobuf - the Go implementation of Google's Protocol Buffers
- protorpc - Google Protocol Buffers RPC for Go and C++
- replican-sync - An rsync algorithm implementation in Go
- Rsync - Rsync algorithm as a Go library
- telnet - Package telnet provides TELNET and TELNETS client and server implementations, for the Go programming language, in a style similar to the "net/http" library (that is part of the Go standard library) including support for "middleware"; TELNETS is secure TELNET, with the TELNET protocol over a secured TLS (or SSL) connection.
- telnet - A simple interface for interacting with Telnet connection
- telnets - A client for the TELNETS (secure TELNET) protocol.
- glibvnc - Go wrapper using CGO for the libvnc library.
- lib/websocket - A library for writing websocket client and server (using epoll)
- Gorilla WebSocket - WebSocket protocol implementation
- websocketd - HTTP server that converts STDIN/STDOUT program into WebSockets service. Also handles HTML and CGI.
- wst - A dead simple WebSocket tester
- ws-cli - WebSocket command line client
- goczmq - Wrapper for the CZMQv3 interface - blog post
- gozmq - Go Bindings for 0mq (zeromq/zmq)
- zmq2 - A Go interface to ZeroMQ (zmq, 0MQ) version 2.
- zmq3 - A Go interface to ZeroMQ (zmq, 0MQ) version 3.
- zmq4 - A Go interface to ZeroMQ (zmq, 0MQ) version 4.
- betwixt - Betwixt implements the OMA Lightweight M2M (LWM2M) protocol for Device Management and Monitoring
- canopus - CoAP Client/Server implementation (RFC 7252)
- chunkedreader - A light weight library for reading continuous fixed sized messages from TCP streams.
- circle - Go interface to the libcircle distributed-queue API
- createsend-go - API client for Monitor http://www.campaignmonitor.com (email campaign service)
- dmrgo - Library for with Hadoop Streaming map/reduce
- doozerconfig - Go package for managing json-encoded configuration in Doozer
- doozerd - A consistent distributed data store
- endless Zero downtime restarts for go servers (Drop in replacement for http.ListenAndServe/TLS)
- gearman-go - A native implementation for Gearman API with Go.
- Glue - Robust Go and Javascript Socket Library (Alternative to Socket.io)
- goagain - zero-downtime restarts in Go
- Go Ajax - Go Ajax is a JSON-RPC implementation designed to create AJAX powered websites.
- gobeanstalk - Go Beanstalkd client library
- go-camo - Go http image proxy (camo clone) to route images through SSL
- go-dbus - A library to connect to the D-bus messaging system
- go-diameter - Diameter stack and Base Protocol (RFC 6733)
- go-smpp - SMPP 3.4 protocol implementation
- go-flowrate - Data transfer rate control (monitoring and limiting)
- gogammu - Library for sending and receiving SMS
- go-icap - ICAP (Internet Content Adaptation Protocol) server library
- gonetbench - Simple TCP benchmarking tool
- gonetcheck - package for checking general internet access
- goodhosts - Simple hosts file (/etc/hosts) management in Go
- gopacket - Packet encoding/decoding, pcap/pfring/afpacket support, TCP assembly, and more!
- gopcap - A simple wrapper around libpcap
- goq - A persistent message queue written in Go.
- goradius - A Radius client written in Go
- go-rpcgen - ProtoBuf RPC binding generator for net/rpc and AppEngine
- gorpc - RPC optimized for high load
- GoRTP - RTP / RTCP stack implementation for Go
- GoSIPs - SIP (Session Initiation Protocol) Stack in Go
- mqtt - MQTT stack in Go
- gosndfile - Go binding for libsndfile
- gosnmp - an SNMP library written in GoLang.
- go-socket.io - A Socket.IO backend implementation written in Go
- gosocks - A SOCKS (SOCKS4, SOCKS4A and SOCKS5) proxy client library in Go.
- go-sslterminator - SSL terminator proxy
- go-statsd-client - Go statsd client library
- Gollum - A n:m multiplexer that gathers messages from different sources and broadcasts them to a set of destinations.
- Grumble - Mumble (VoIP) server implementation
- handlersocket-go - Go native library to connect to HandlerSocket interface of InnoDB tables
- HomeControl - an implementation of Apple's HomeKit Accessory Protocol (HAP)
- Hprose - Hprose is a High Performance Remote Object Service Engine.
- httpfstream - streaming append and follow of HTTP resources (using WebSockets)
- ipaddress - Convenient ip address functions: ip -> int, int -> ip, and IPNet broadcast address
- iris-go - Go binding for the Iris decentralized messaging framework.
- iris - Peer-to-peer messaging for back-end decentralization.
- kafka.go - Producer & Consumer for the Kafka messaging system
- lcvpn - Decentralized VPN implementation
- ldap - Basic LDAP v3 functionality for the GO programming language.
- mbxchan - An easy communication between distributed Go applications using standard Go channels and remote procedure calls.
- nagiosplugin - package for writing Nagios/monitoring plugins
- NATS - NATS distributed messaging system client for Go
- netsnail - A low-bandwidth simulator
- netutils - Simple interface for turning TCP Sockets into channels.
- npipe - a pure Go wrapper for Windows named pipes
- norm - reliable UDP using multicast and unicast sockets
- opendap - Go wrapper for Openldap
- pusher-http-go - Go library for interacting with the Pusher Realtime API
- QRP - QRP is a simple packet-based RPC protocol designed as a simple alternative to Go's rpc, that can run over UDP
- remotize - A remotize package and command that helps remotizing methods without having to chaneg their signatures for rpc
- Resgate - A Realtime + REST API Gateway for NATS to create web APIs with live data
- rs232 - Serial interface for those of us who still have modems (or arduinos)
- rss - RSS parsing library.
- seamless - Reverse TCP Proxy with HTTP management API
- shell2http - Executing shell commands via simple http server
- sockjs-go - Implements server side counterpart for the SockJS-client browser library.
- SOCKS5 Server - Scalable SOCKS5 server with Access Control Lists
- spark - Emergency web server (for static files)
- spdy - SPDY library, wired into net/http, currently supporting servers only.
- statsd-go - Statsd implementation in Go, forked from gographite, which submits to Ganglia
- stompngo_examples - Examples for stompngo.
- stompngo - A Stomp 1.1 Compliant Client
- tcp_fallback - A TCP proxy implementing a simple fallback mechanism.
- tcpmeter - A TCP throughput measuring tool
- toxiproxy - Framework for simulating network conditions.
- traceroute - A traceroute implementation
- traefik - Modern, reverse proxy in Go
- Uniqush - A free and open source software which provides a unified push service for server-side notification to apps on mobile devices.
- uritemplates - A level 4 implementation of URI Templates (RFC 6570)
- VDED - Vector Delta Engine Daemon - track deltas in ever-increasing values (written in Go)
- zero-downtime-daemon - Configurable zero downtime daemon (Hot Update) framework for any kind of TCP,HTTP,FCGI services
- zeroupgrade - Upgrades network servers with zero downtime
- cwmp-proxy - Reverse cwmp proxy
- netstat-nat - Display NAT entries on Linux systems
- go-nat-pmp - A client for the NAT-PMP protocol used in Apple and open-source routers
- humanize-bytes - Command-line utilities to convert "MiB" etc to raw numbers, and back
- Go FUSE file system library - From-scratch implementation of the kernel-userspace communication protocol based on Russ Cox'.
- Go-fuse - Library to write FUSE filesystems in Go
- go-osx-xattr - Package xattr wraps OS X functions to manipulate the extended attributes of a file, directory and symbolic link.
- inspect/os - Metrics library for operating system measurements (Linux/MacOSX)
- service - Service will install / un-install, start / stop, and run a program as a service (daemon) on Windows/Linux and OSX.
- go-nbd - Library to write block devices for Linux in Go.
- goconc - A collection of useful concurrency idioms and functions for Go, compiled
- go-crazy - An experimental source-to-source compiler for go
- go-gtk-demo - A demonstration of how to use GTK+ with Go.
- go-hashmap - A hash table in pure go as an experiment in Go performance
- golang-examples - A bunch of golang examples
- GolangSortingVisualization - A visualization of various sorting algorithms in Go
- golibs - A collection of tiny go packages (and also a test repo for various CI and coverage services)
- goplay - A bunch of random small programs in Go
- lifegame-on-golang - Game of Life in Go
- linear - Playing around with the linear algebra
- pl0 - PL/0 front end, compiler and VM. .
- project euler in go - Solutions to Project Euler in Go also
- shadergo - shader test using Go
- travisci-golang-example - Travis-CI example for Go
- DHT - Kademlia DHT node used by Taipei-Torrent, compatible with BitTorrent
- ed2kcrawler - eDonkey2000 link crawler
- gop2p - A simple p2p app to learn Go
- go-p2p - P2P module for blockchains and more
- GoTella - A Go implementation of the Gnutella Protocol
- Rain - BitTorrent client and library
- Taipei-Torrent - A BitTorrent client
- Tendermint - P2P Byzantine-Fault-Tolerant consensus & blockchain stack
- wgo - A simple BitTorrent client based in part on the Taipei-Torrent and gobit code
- DHT - BitTorrent DHT Protocol && DHT Spider.
- go-slices - Helper functions for manipulating slices in Go just like the official "strings" package provides
- go-clang - cgo bindings to the C-API of libclang
- godeferred - port of jsdeferred: http://cho45.stfuawsc.com/jsdeferred/
- go-galib - a library of Genetic Algorithms
- go-intset - a library to work with bounded sets of integers, including multiple alternative implementations
- go-parse - a Parsec-like parsing library
- sh - a shell/bash parser and formatter
- Shuffle - Implementation of the Fisher–Yates shuffle (or Knuth shuffle) in Go.
- fileb0x - Simple tool to embed files in go with focus on "customization" and ease to use.
- go-bindata - Package that converts any file into managable Go source code.
- go-resources - Unfancy resources embedding with Go.
- go.rice - go.rice is a Go package that makes working with resources such as html,js,css,images and templates very easy.
- implant - implant allows embedding static resources, from a series of directories (recursively).
- statics - Embeds static resources into go files for single binary compilation + works with http.FileSystem + symlinks.
- ebnf2y - Utility for converting EBNF grammars into yacc compatible skeleton .y files.
- gocc - Go Compiler Compiler
- golex - Lex/flex like fast (DFA) scanners generator.
- gopp - Go Parser Parser
- goyacc - Goyacc is a version of yacc generating Go parsers.
- y - Package y converts .y (yacc) source files to data suitable for a parser generator.
- yy - yacc to yacc compiler.
- flexgo - A version of flex that can produce Go code.
- fsm - FSM (NFA, DFA) utilities.
- Ragel - State Machine Compiler
- lexmachine - Lexical Analysis Framework for Golang
- acme - ACME certificate acquisition tool
- acra - SQL database protection suite: strong selective encryption, SQL injections prevention, intrusion detection system
- casbin - An authorization library that supports access control models like MAC, RBAC, ABAC
- docker-slim - Container security and optimization
- gryffin - A large scale security scanner by Yahoo!
- hyperfox - a security tool for proxying and recording HTTP and HTTPs communications on a LAN
- lego - Let's Encrypt client and ACME library
- webseclab - a sample set of web security test cases and a toolkit to construct new ones
- godes - Library for building discrete event simulation models
- funnelsort - Lazy funnel sort -- a cache-oblivious sorting algorithm
- Sortutil - Nested, case-insensitive, and reverse sorting for Go.
- sortutil - Utilities supplemental to the Go standard "sort" package
- tarjan - Graph loop detection function based on Tarjan's algorithm
- timsort - Fast, stable sort, uses external comparator or sort.Interface
- bubble-sort - minimal implementation of the bubble-sort algorithm
- cocktail-shaker-sort - minimal implementation of the cocktail-shaker-sort algorithm
- Gitfile - A lightweight package manager for installing git repos
- go-deps - Analyzes and recursively installs Go package deps (library functionality similar to
go get
) - go-diff - A diff command for go languange showing semantic differences of two go source files.
- gogitver - Embeds a git tag (version string) into your application
- go-many-git - Manage and run commands across multiple git repositories
- go-pkgs - Finds all matching packages in all of the GOPATH trees (library functionality similar to
go list all
) - go-vcs - clone and check out revs of VCS repositories (git and hg support)
- go-vcsurl - Lenient VCS repository URL parsing library
- hggofmt - A Mercurial/hg extension with a hook to
- nut - Nut is a tool to manage versioned Go source code packages, called "nuts".
- vcstool - VCS abstraction tool
- Minio - Object Storage compatible with Amazon S3 API
- libStorage - an open source, platform agnostic, storage provisioning and orchestration framework, model, and API
- OpenEBS - Containerized, Open source block storage for your containers,integrated tightly into K8S and other environments and based on distributed block storage and containerization of storage control
- storage - An application-oriented unified storage layer for Golang
- allot - Placeholder and wildcard text parsing for CLI tools and bots
- awk - Easy AWK-style text processing in Go
- binarydist - Binary diff and patch
- Black Friday - A markdown processor
- codename-generator - A codename generator meant for naming software releases
- columnize - format slice or array into aligned columns
- csvplus - Extends the standard Go encoding/csv package with fluent interface, lazy stream operations, indices and joins.
- csvutil - A heavy duty CSV reading and writing library.
- dgohash - Collection of string hashing functions, including Murmur3 and others
- douceur - A simple CSS parser and inliner in Go.
- dsv - A library for working with delimited separated value (DSV).
- flux - Fluent Regular Expressions in golang
- genex - Expansion of Regular Expressions
- gettext-go - GNU's gettext support, written in pure Go
- gettext - Golang bindings for gettext; Feature complete, cgo
- go-colortext - Change the color of the text and background in the console, working both in Windows and other systems.
- go-guess - Go wrapper for libguess
- goagrep - fast fuzzy string matching using precomputation
- goini - A go library to parse INI files
- golorem - lorem ipsum generator
- go-migemo - migemo extension for go (Japanese incremental text search)
- go-ngram N-gram index for Go
- goregen - A Go library for generating random strings from regular expressions.
- goskirt - Upskirt markdown library bindings for Go
- gosphinx - A Go client interface to the Sphinx standalone full-text search engine
- govalidator - Package of string validators and sanitizers
- gpKMP - String-matching in Go using the Knuth–Morris–Pratt algorithm
- hangul - Handy tools to manipulate Korean character
- html2text - Golang HTML to text conversion library
- intern - Map strings to symbols for constant-time comparisons
- kasia.go - Templating system for HTML and other text documents
- kview - Simple wrapper for kasia.go templates. It helps to modularize content of a website
- liquid - A complete implementation of Shopify Liquid templates
- logparse - Parser for most common log formats
- NTemplate - Nested Templates
- parse - PEG parser that uses reflection to define grammar
- peg - Parsing Expression Grammer Parser
- pigeon - Parsing Expression Grammar (PEG) Parser generator for Go
- plural - No-fuss plurals for formatting both countable and continuous ranges of values.
- polyglot - String translation utilities for Go
- pretty.go - Pretty-printing for go values
- raymond - A complete handlebars implementation in Go.
- rubex - A simple regular expression library that supports Ruby's regex syntax. It is faster than Regexp.
- sanitize - Package sanitize provides functions for sanitizing html and text.
- scanner - A text scanner that parses primitive types, analogous to Java's
- segment - An implementation of Norvig's recursive word segmentation algorithm
- sprig - Template functions for Go templates.
- strftime - strftime implementation
- strit - Package strit introduces a new type of string iterator, as well as a number of iterator constructors, wrappers and combinators.
- strogonoff - Stenography with Go
- strutil - Package strutil collects utils supplemental to the standard strings package.
- text - Text paragraph wrapping and formatting
- Tideland golib - Stringex package for statistcal analysis
- TySug - Alternative suggestions with respect to keyboard layouts.
- useragent - User agent string parser
- xurls - Extract urls from text
- assert - Basic Assertion Library used along side native go testing, with building blocks for custom assertions
- assert - Assert for go test.
- assert - Handy assert package.
- assert - JUnit-like asserts with excellent error messages
- biff - Bifurcation testing framework, BDD compatible.
- charlatan - Tool to generate fake interface implementations for tests.
- conex - Docker containers for integration tests
- counterfeiter - Tool for generating self-contained and type-safe mocks.
- downtest - Automatically run tests for all known downstream consumers of a Go package.
- ginkgo - BDD Testing Framework for Go.
- go2xunit - Convert "go test -v" output to xunit XML output
- go-assert - Testing utils for Go.
- goautotest - Automatically run unit tests when code changes are made
- goblin - Minimal and Beautiful Go testing framework
- Gocheck - Rich test framework with suites, fixtures, assertions, good error reporting, etc
- GoConvey - Browser-based reporting, uses
go test
, supports traditional Go tests, clean DSL - gocov - Code coverage testing/analysis tool
- gomega - Ginkgo's Preferred Matcher Library.
- gomock - a mocking framework for Go.
- GoSpec - a BDD framework
- gospecify - another BDD framework
- go-stat - performant instrumentation/profiling for Go
- go-tap - TAP (Test Anything Protocol) parser in Go
- go-testdeep - Extremely flexible deep comparison, extends the testing package
- gotest.tools - a collection of packages for writing readable tests
- gotestsum - a test runner with customizable and colored output
- gounit - xunit for Go
- GSpec - Expressive, reliable, concurrent and extensible Go test framework that makes it productive to organize and verify the mind model of software.
- httpexpect - Concise, declarative, and easy to use end-to-end HTTP and REST API testing
- Nitro - A quick and simple profiler For Go
- mspec - BDD framework that frees you to Stub and Spec your code first with natural BDD language.
- muxy - Simulating real-world distributed system failures.
- Pegomock - a mocking framework based on golang/mock, but uses a DSL closely related to Mockito.
- rapid - property-based testing library with integrated shrinking
- terst - A terse, easy-to-use testing library for Go
- test2doc - Generate documentation for your go units from your unit tests.
- testfixtures - Rails' like test fixtures for testing database driven apps.
- testflight - Painless http testing in Go
- Testify - A set of packages that provide many tools for testifying that your code will behave as you intend.
- ut - Awesome mocks without magic.
- make.go.mock - Generates type-safe mocks for Go interfaces and functions.
code.soquee.net/testlog
— Alog.Logger
that proxies to the Log function on a testing.T so that logging only shows up on tests that failed, grouped under the test.
- validator - Go Struct and Field validation, including Cross Field, Cross Struct, Map, Slice and Array diving
- validation - Simple independent struct/key-value validation
- git (in go) - Minimal working git client in Go
- gogs - Self-hosting Git Server in Go
- semver - Semantic Versioning (SemVer) library
- agora - A dynamically typed, garbage collected, embeddable programming language built with Go
- anko - Scriptable interpreter written in golang
- evalfilter - Embedded evaluation engine for filtering objects.
- expr - Expression evaluator with static typing
- forego - Forth virtual machine
- Gelo - Extensible, embeddable interpreter
- Gentee - Embeddable scripting programming language
- GoAwk - An implementation of awk in golang.
- GoBASIC - An embeddable BASIC interpreter written in golang.
- GoEmPHP - This package is built for Embedding PHP into Go
- Goja - ECMAScript 5.1(+) implementation written in Go (otto fork with byte code compiler)
- goenv - Create an isolated environment where you install Go packages, binaries, or even C libraries. Very similar to virtualenv for Python.
- GoForth - A simple Forth parser
- golemon - A port of the Lemon parser-generator
- golem - A general purpose, interpreted scripting language.
- GoLightly - A flexible and lightweight virtual machine with runtime-configurable instruction set
- goll1e - An LL(1) parser generator for the Go programming language.
- Golog - Prolog interpreter in Go
- go-lua - Shopify's lua interpreter
- golua - A fork of GoLua that works on current releases of Go
- gomruby - mruby (mini Ruby) bindings for Go
- gopher-lua - A Lua 5.1 VM and compiler written in Go
- go-php - PHP bindings for Go
- go-python - go bindings for CPython C-API
- gotcl - Tcl interpreter in Go
- go.vm - Simple virtual machine which interprets bytecode.
- v8 - V8 JavaScript engine bindings for Go (supports V8 builds at least up to 5.8.244)
- go-v8 - V8 JavaScript engine bindings for Go
- Hivemind - Process manager for Procfile-based applications
- LispEx - A dialect of Lisp extended to support for concurrent programming, written in Go.
- Minima - A language implemented in Go
- ngaro - A ngaro virtual machine to run retroForth images
- otto - A JavaScript parser and interpreter written natively in Go
- Overmind - Process manager for Procfile-based applications and tmux
- RubyGoLightly - An experimental port of TinyRb to Go
- Yaegi - A complete Go interpreter in Go
- Presento - The simplest possible cross-platform remote control for the presentations
- Caddy - A fast, capable, general-purpose HTTP/2 web server that's easy to use
- Dataflowkit - Web scraping Service to turn websites into structured data.
- Digestw - A Web Application - Twitter's Timeline Digest
- fabio - A fast zero-conf load balancing HTTP router for microservices.
- Filestash - A web client for SFTP, S3, FTP, WebDAV, Git, Minio, LDAP, Caldav, Carddav, Mysql, Backblaze, ...
- fourohfourfound - A fallback HTTP server that may redirect requests with runtime configurable redirections
- Fragmenta - A CMS built in Go
- freegeoip - IP geolocation web service (web server of freegeoip.net)
- goals-calendar - A web-based Seinfeld calendar implemented in Go
- goblog - A static blog engine
- gocrawl - A polite, slim and concurrent web crawler.
- goflash - Flash player implementation in Go language
- gogallery - simple web server with an emphasis on easily browsing images
- gojekyll - A golang clone of the Jekyll static site generator
- goof - A simple http server to exchange files over http (upload/download)
- gopages - A php-like web framework that allows embedding Go code in web pages
- go_spider - A flexible ,modularization and concurrent web crawler framework.
- GoURLShortener - A frontend for the http://is.gd/ URL shortener
- gowall - A website and user system
- httpfolder - A http server to exchange files over http with basic authentication (upload/download)
- Hugo - A fast and flexible static site generator implemented in Go
- Já Vai Tarde - Unfollows monitoring for Twitter
- kurz.go - a url shortener based on web.go and redis
- Monsti - Resource friendly flat file CMS for private and small business sites.
- now.go - A simple HTTP-based to-do queue.
- Peach - A web server for multi-language, real-time synced and searchable documentation.
- rabbitmq-http - REST API for RabbitMQ
- grabbit - A lightweight transactional message bus on top of RabbitMQ
- serve-files - Far-future and gzip wrapper around the standard net/http server.
- sf_server - a tiny send file server and client
- SuperSaaS API Client - HTTP client library for the supersaas.com scheduling/bookings/appointments API
- Tideland golib - Web package for REST request handling
- Vantaa - A modular blogging API engine written in Go, Neo4j and Polymer.
- websiteskeleton - Simple net/http website skeleton
- webtf - Web app to graphical visualization of twitter timelines using the HTML5
- Wikifeat - Extensible wiki system using CouchDB written in Golang
- Freyr - Server for storing and serving readings from plant environment sensors. Integrates Golang API with ReactJS web app; uses Docker for testing/deployment.
- Rickover - Job queue with a HTTP API, backed by Postgres
- goth - Package goth provides a simple, clean, and idiomatic way to write authentication packages for Go web applications
- authcookie - Package authcookie implements creation and verification of signed authentication cookies.
- dgoogauth - Go port of Google's Authenticator library for one-time passwords
- goauth - A library for header-based OAuth over HTTP or HTTPS.
- GOAuth - OAuth Consumer
- go-http-auth - HTTP Basic and HTTP Digest authentication
- Go-OAuth - OAuth 1.0 client
- goha - Basic and Digest HTTP Authentication for Go http client
- hero - OAuth server implementation - be an OAuth provider with Go
- httpauth-go - Package httpauth provides utilities to support HTTP authentication policies. Support for both the basic authentication scheme and the digest authentication scheme are provided.
- httpauth - HTTP session (cookie) based authentication and authorization
- oauth1a - OAuth 1.0 client library
- OAuth Consumer - OAuth 1.0 consumer implementation
- otp - HOTP and TOTP library with command line replacement for Google Authenticator
- securecookie - Encode and Decode secure cookies
- totp - Time-Based One-Time Password Algorithm, specified in RFC 6238, works with Google Authenticator
- go-otp - Package go-otp implements one-time-password generators used in 2-factor authentication systems like RSA-tokens. Currently this supports both HOTP (RFC-4226), TOTP (RFC-6238) and Base32 encoding (RFC-3548) for Google Authenticator compatibility
code.soquee.net/otp
— A library for generating one-time passwords using HOTP (RFC-4226), and TOTP (RFC-6238). Includes less commonly used profiles, and custom time functions for flexible windows.
- Cascadia - CSS selector library
- GoQuery - jQuery-like DOM manipulation library, using Go's experimental HTML package.
- goq - jQuery-like declarative struct tag scraping and unmarshaling based on GoQuery.
- html-query - A fluent and functional approach to querying HTML.
- HTML Transform - A CSS selector based html scraping and transformation library
- aah - A scalable, performant, rapid development Web framework for Go.
- Aero - Fast and secure web server for Go.
- Air - An ideal RESTful web framework for Go.
- alien - A lightweight and fast http router
- app.go - Web framework for google app engine
- arasu - A Lightning Fast Web Framework written in Go & Dart
- Beego - Beego is an open source version of the scalable, non-blocking web framework.
- browserspeak - Generate HTML templates, CSS or SVG without writing
<
or>
- erouter - The erouter is a slightly less scalable and feature-rich scalable router than httprouter.
- eudore - Eudore is a high performance and easy to extend open source golang web framework.
- httpcoala - Library for request coalescing - handy for reverse proxies.
- falcore - Modular HTTP server framework
- fcgi_client - Go fastcgi client with fcgi params support
- Flamingo Framework - Framework for building pluggable production ready web projects.
- florest - High-performance workflow based REST API framework in Go
- forgery - A clone of the superb Node.js web framework Express.
- gramework - The truly fastest web framework for Go. Battle tested, highly effective baseline for your web apps.
- Gin Web Framework - Martini-like API and httprouter gives it good performance.
- Goal - A toolkit for high productivity web development in Go language built around the concept of code generation.
- Go-Blog - Blog framework written in Go
- go-fastweb - aims to be a simple, small and clean MVC framework for go
- goku - a Web Mvc Framework for Go, mostly like ASP.NET MVC.
- Golanger - Golanger Web Framework is a lightweight framework for writing web applications in Go.
- Goldorak.Go - a web miniframework built using mustache.go, web.go and Go-Redis
- go-restful - lean package for building REST-style Web Services
- GoRest - An extensive configuration(tags) based RESTful style web-services framework.
- go-rest - A small and evil REST framework for Go
- gorilla - Gorilla web toolkit
- GoSrv - A Go HTTP server that provides simple command line functionality, config loading, request logging, graceful connection shutdown, and daemonization.
- go-start - A high level web-framework for Go
- go-urlshortener - interface to google's urlshorten API
- goweb - Lightweight RESTful web framework for Go providing Ruby on Rails style routing
- go-webproject - Modular web application framework and app server
- Gowut - Go Web UI Toolkit is a full-featured, easy to use, platform independent Web UI Toolkit written in pure Go.
- Goyave - An elegant, full-featured web application framework
- HttpRouter - A high performance HTTP request router that scales well
- limiter - Simple rate limter middleware for Go
- Macaron - Modular web framework in Go
- mango - Mango is a modular web-application framework for Go, inspired by Rack, and PEP333.
- Martini deprecated - Martini is a popular, lightweight, extensible package for writing modular web apps/services in Go
- Negroni - Idiomatic middleware for Go
- restclient - Client library for interacting with RESTful APIs.
- resty - REST client library inspired by Ruby rest-client.
- Revel - High productivity web framework modeled on Play! Framework
- Ringo - Lighweight MVC web framework inspired by Rails, Gin.
- sawsij - Provides a small, opinionated web framework.
- Tango - Micro-kernel & pluggable web framework for Go
- Tiger Tonic - framework for building JSON web services inspired by Dropwizard
- trinity - MVC framework
- uAdmin - Web framework with a back end GUI similar to Django.
- Utron - MVC Framework
- Violetear - HTTP router
- web.go - a simple framework to write webapps
- wfdr - Simple web framework designed for and written in go. Works with other languages as well, but not as well.
- xweb - A web framework for Go. Just like Struts for Java.
- form - Complete bidirectional HTML form encoder & decoder (x-www-form-urlencoded) for arbitrary data (package encoding compatible)
- gforms - HTML forms for Go
- Go-FORM-it - Go library for easy and highly-customizable forms creation and template rendering.
- GoForms - Form data validation, cleaning and error reporting - a la django.forms
- htmlfiller - Fills in html forms with default values and errors a la Ian Bicking's htmlfill for Python
- MonstiForm - HTML form generator and validator library
- revel-csrf - Cross-Site Request Forgery (CSRF) attacks prevention for the Revel framework
- xsrftoken - A package for generating and validating tokens used in preventing XSRF attacks
- adn - Interface to the App.net API
- anaconda - Client library for the Twitter 1.1 API
- ddg - DuckDuckGo API interface
- facebook - Up-to-date facebook graph API client. Handy and flexible
- filepicker-go - Go library for the Filepicker's REST API
- firebase - Client library for the Firebase REST API
- gh - Scriptable server and net/http middleware for GitHub Webhooks API
- github - Go library for accessing the GitHub REST API v3
- githubql - Go library for accessing the GitHub GraphQL API v4
- gobo - Client library for Sina Weibo
- gocaptcha - gocaptcha provides easy access to the reCaptcha API in go
- go-dealmap - Go library for accessing TheDealMap's API
- go-dropbox - API library for dropbox
- go-flickr - A wrapper for Flickr's API
- go-get-youtube - A simple library+client for fetching meta data of, and downloading Youtube videos
- go-gravatar - Wrapper for the Gravatar API
- go-hummingbird - Go library for accessing the Hummingbird.me API
- go-libGeoIP - GO Lib GeoIP API for Maxmind
- gominatim - Go library to access nominatim geocoding services
- gomojo - Instamojo API wrapper
- gomwapi - Access mediawiki contents like wikipedia, wiktionary in golang
- go-myanimelist - Go library for accessing the MyAnimeList API
- googtrans - unofficial go bindings for Google Translate API v2
- go-recaptcha - Handles reCaptcha form submissions in Go
- gorecurly - A Client app to use with Recurly's api
- go.strava - Official client library for the Strava V3 API
- go.stripe - a simple credit card processing library for Go using the Stripe API
- Gotank - Searchify's Go client for the IndexTank full-text search API
- go-tripit - Go API library for the TripIt web services
- GoTwilio - Twilio library for Go (golang). Very basic at the moment
- gravatar - Gravatar image/profile API library
- jsonapi - Generate JSON API from Go structs
- postmark - Access postmark API from Go
- reddit.go - Client library for Reddit API
- shorturl - Generic implementation for interacting with various URL shortening services
- Stack on Go - Go wrapper for Stack Exchange API
- stripe - Official Stripe client library
- SocialSharesCount - Wrapper API on multiple social websites to get URL share statistics
- twilio - Simple Twilio API wrapper
- twittergo - Client library for Twitter's API
- cloudcomb-go-sdk - Go client library for CloudComb
- adhoc-http - Quick & dirty HTTP static file server
- assets - Helps prepares CSS and JS files for development and production of Go web apps.
- bwl - a set of libraries to help build web sites
- captcha - Image and audio captcha generator and server
- gaerecords - Lightweight wrapper around appengine/datastore providing Active Record and DBO style management of data
- gcd - provides helpful functions to work with Google Cloud DataStore.
- get2ch-go - a library to access the 2channel Japanese web bulletin board
- gofeed - Parse RSS and Atom feeds in Go
- go-gzip-file-server - A net.http.Handler similar to FileServer that serves gzipped content
- gohaml - An implementation of the popular XHTML Abstraction Markup Language using the Go language.
- go-httpclient - a Go HTTP client with timeouts
- gojwt - Json Web Tokens for Go
- go-pkg-rss - a packages that reads RSS and Atom feeds
- gorefit - A library for theming existing websites
- goreman - foreman clone
- GoRequest - Simplified HTTP client with rich features such as proxy, timeout, and etc. ( inspired by nodejs SuperAgent )
- goroute - A very simple URL router based on named submatches of regular expression that works well with http.Handler .
- gorouter - Simple router for go to process url variables
- go-rss - Simple RSS parser and generator
- go-rss - Simple RSS parser, tested with Wordpress feeds.
- goscribble - An MPD Audioscrobble
- go-twitter - another Twitter client
- go-twitter-oauth - a simple Twitter client (supports OAuth)
- grender - Go static site generator
- halgo - HAL-compliant API client and serialisation library.
- http-gonsole - Speak HTTP like a local. (the simple, intuitive HTTP console, golang version)
- httprpc - HTTP RPC codecs (json2, soap, rest)
- HypeCMS - A flexible CMS built with Go and MongoDb.
- Kontl - A client for kon.tl's URL shortening service
- mustache.go - an implementation of the Mustache template language
- muxer - Simple muxer for a Go app without regexp
- Optimus Sitemap Generator - A universal XML sitemap generator
- passwordreset - Creation and verification of secure tokens useful for implementation of "reset forgotten password" feature in web applications.
- pat - A Sinatra style pattern muxer
- persona - remote verification API for persona
- plex - simple, small, light, regexp http muxer with chaining
- purell - tiny Go library to normalize URLs
- pusher.go - HTTP Server Push module for the standard http package
- rest2go - Based on rest.go, forked for improvements and REST consistency
- rest.go (forked) - forked rest.go for improvements and REST consistency
- resty - Simple HTTP and REST client for Go inspired by Ruby rest-client.
- robotstxt - The robots.txt exclusion protocol implementation. Allows to parse and query robots.txt file.
- scs - A HTTP session manager using server-side sessions stores.
- seshcookie - A web session library inspired by Beaker
- soy - A Go implementation for Soy templates (Google Closure templates). High performance and i18n.
- user_agent - An HTTP User-Agent parser
- webtestutil - Web and HTTP functional testing utilities. Includes Gorilla testing support.
- aop - Aspect Oriented Programming For Go.
- yt2pod - Daemon that monitors YouTube channels and publishes audio podcasts of them
- gform - An easy to use Windows GUI toolkit for Go
- go-ole - win32 ole implementation for golang
- go-Windows-begin - for the absolute Windows-Go beginner
- w32 - Windows API wrapper for Go.
- walk - "Windows Application Library Kit" for the Go Programming Language
- Windows Command Line Shutdown - A tool to shutdown Windows Computer from Command Prompt
- inspect - Linux and MacOSX systems monitoring and diagnostics
- unixsums - Legacy Unix checksums: cksum, sum
The following entries have not been filed. Please help by putting these in relevant categories.
- GoBot - PoC Go HTTP Botnet
- ebml-go - EBML decoder
- go-bindata - Converts any file into manageable Go source code for embedding binary data into a Go program.
- consistent - Consistent hashing with bounded loads.
- goconsistenthash - Consistent hashing library (based on http://www.lexemetech.com/2007/11/consistent-hashing.html)
- go-cron - A small cron job system to handle scheduled tasks, such as optimizing databases or kicking idle users from chat. The cron.go project was renamed to this for
go get
compatibility. - godebiancontrol - Golang debian control file parser
- go-gmetric - Ganglia gmetric protocol support
- gographviz - Graphviz DOT language parser for golang
- godotviz - Rendering graphics files from "DOT language". Written in golang
- dotviz-server - WebBased DOT language visualization tool written in go
- goseq - command line tool, written in Go, for creating sequence diagrams using a text-based description language.
- golor - golor is a command line tool for golang source code coloring
- gopcapreader - Presents realtime pcap data as io.Reader objects
- go.psl - Go regdom-libs/public suffix list
- go-webfinger - Simple Client Implementation of WebFinger
- img-LinuxFr.org - A reverse-proxy cache for external images used on LinuxFr.org
- seed - Easily seed PRNGs with some entropy
- spellabc - Package spellabc implements spelling alphabet code word encoding.
- Twackup - Backs up your tweets into local files
- Tasks - A simplistic todo list manager written in Go
- one-file-pdf - A minimalist Go PDF writer in <2K lines and 1 file